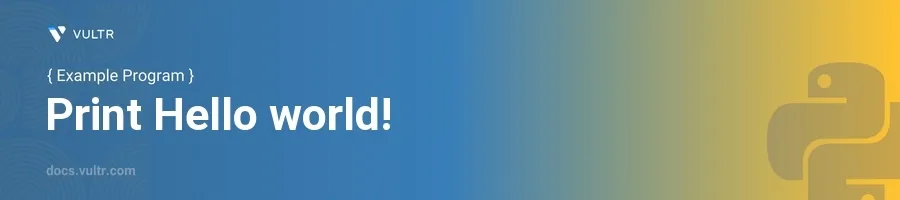
Introduction
The phrase "Hello world!" is renowned in the programming community as the traditional first code that many learners write when exploring a new programming language. This simple example helps new programmers understand basic syntax and the process of writing and running a program. For Python, which is known for its simplicity and readability, writing a "Hello world!" program is exceptionally straightforward.
In this article, you will learn how to display the "Hello world!" message in Python using various methods. Each method will be illustrated with example code, helping you grasp different ways Python can handle this simple output task.
Basic "Hello world!" Program
Using the print() Function
Open your Python integrated development environment (IDE) or any text editor.
Type the following Python code:
pythonprint("Hello world!")
Save the file with a
.py
extension, for example,hello.py
.Run the script in your IDE or from the command line.
To execute the script from the command line, navigate to the directory containing your script and run:
consolepython hello.py
This code simply uses Python's built-in
print()
function to send the string "Hello world!" to the console.
Adding a Comment
It’s good practice to comment your code. Add a comment above the print statement.
python# Printing Hello world! to the console print("Hello world!")
Save and run the file as you did before.
Including comments in your code helps other developers, and your future self, understand what the code is supposed to do. Here, though obvious, the comment indicates that the line prints a message to the console.
Advanced Printing Methods
Using a Main Function
In Python, defining a main function to encapsulate the execution logic of your script is a good practice, especially as your projects grow larger.
Here’s how you organize the "Hello world!" script with a main function:
pythondef main(): print("Hello world!") if __name__ == "__main__": main()
Save and run your script as previously described.
This script defines a
main()
function, which is a common pattern in Python programs. Theif __name__ == "__main__":
check ensures thatmain()
runs only if the script is executed as the main program.
Formatting with f-Strings (Python 3.6+)
Python 3.6 introduced f-Strings for a more convenient way to embed expressions inside string literals. Here's how you can use them:
Modify the
print()
function to use an f-String:pythonmessage = "Hello world!" print(f"{message}")
Save and execute your script.
F-strings provide a way to embed expressions inside string literals using minimal syntax. It makes the code more readable and concise.
Conclusion
Printing "Hello world!" in Python is not just a tradition but also a great way to start familiarizing yourself with the syntax and execution of Python scripts. From using the basic print()
function to embedding a message in f-strings, these methods represent just a few ways to accomplish this task. Recognizing these different approaches can help you understand the flexibility of Python as a programming language. Explore each method to keep your foundational Python skills sharp and effective.
No comments yet.