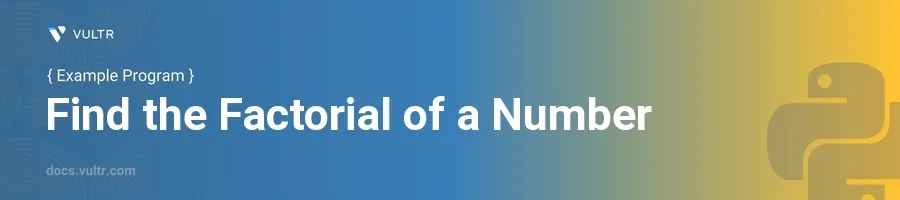
Introduction
The factorial of a number is a mathematical operation that multiplies a given number by every positive integer less than itself. For example, the factorial of 5 (denoted as 5!) is calculated as 5 * 4 * 3 * 2 * 1, which results in 120. Factorials are commonly used in permutations, combinations, and other statistical calculations. In programming, calculating the factorial of a number is a common task utilized to demonstrate the implementation of recursion and iteration.
In this article, you will learn how to write Python programs to find the factorial of a number using different methods. Each method is supplemented with examples to ensure you understand how to implement them effectively in your coding projects.
Using Iteration to Calculate Factorial
Employ a For Loop
Initialize a result variable to 1 since the factorial of 0 is 1.
Loop through numbers from 1 up to and including the target number.
Multiply the result by each number in the loop.
pythondef factorial_iterative(n): result = 1 for i in range(1, n + 1): result *= i return result
This function takes an integer
n
and computes its factorial using a for loop. In each iteration,result
is multiplied by the current value ofi
, which progresses from 1 ton
.
Using While Loop
Initialize the variables: set the result to 1 and start from 1.
Use a while loop to multiply the result until the number equals the factorial number.
In each iteration, increment the number by 1.
pythondef factorial_while(n): result = 1 number = 1 while number <= n: result *= number number += 1 return result
Here, the
while
loop continues running as long asnumber
is less than or equal ton
. During each pass of the loop,result
is multiplied bynumber
, and thennumber
is incremented.
Implementing Recursive Factorial Calculation
Define a function that calls itself to calculate the factorial.
Establish a termination condition where if
n
is 0 or 1, the function returns 1.Otherwise, recursively call the function with
n-1
and multiply the result byn
.pythondef factorial_recursive(n): if n in (0, 1): # base case return 1 return n * factorial_recursive(n - 1)
Recursive functions are powerful for tasks like finding factorial because the factorial computation follows the definition of recursion (factorial(n) = n * factorial(n-1)). The function calls itself with decreasing values of
n
until it reaches the base case.
Utilizing Python's Math Module
Python’s standard library includes a function for computing factorial directly.
Import the
factorial
function from themath
module.Pass the desired number to the
factorial
function.pythonfrom math import factorial result = factorial(5) print(result)
The
math
module’sfactorial
function is optimized and concise, making it an excellent choice when you don't need to implement the function manually for educational purposes. It handles large numbers efficiently and is straightforward to use.
Conclusion
Writing a Python program to find the factorial of a number introduces you to fundamental programming concepts such as loops, recursion, and the use of built-in functions. By following the methods described and utilizing the examples given, you can confidently implement factorial calculation in any Python project. Whether for mathematical applications or as a component of algorithmic challenges, these techniques ensure your programs are efficient and effective. Remember, while the recursive method offers a direct approach matching the mathematical definition, iterative and using Python’s built-in library can provide more performance stability with large numbers.
No comments yet.