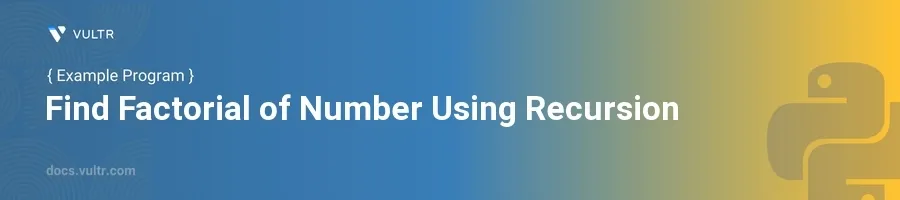
Introduction
A factorial of a non-negative integer is the product of all positive integers less than or equal to that integer. For example, the factorial of 5 is 120
(calculated as 5×4×3×2×1). Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. In Python, recursion can be a natural method to compute factorials due to its mathematical definition that lends itself well to recursive thinking.
In this article, you will learn how to implement a Python program to find the factorial of a number using recursion. You'll see how to define a recursive function, explore its use through examples, and understand how the recursive call stack operates for factorials.
Understanding the Recursive Factorial Function
Defining a Recursive Function
To understand recursion in the context of calculating factorials, start by defining a recursive function named
factorial
.If the input number
n
is0
, the factorial should return1
, since the factorial of zero is defined as1
.If
n
is greater than0
, the function should returnn
multiplied by the factorial ofn-1
.pythondef factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
In this function:
- If
n
is 0, it directly returns1
. - If not, the function calls itself with the argument
n-1
, effectively reducing the problem size with each call until it reaches 0.
- If
Applying the Recursive Function
To find the factorial of a number using the
factorial
function, simply call it with a positive integer.It is advisable to check if the passed number is a non-negative integer to prevent errors from incorrect inputs.
pythonnumber = 5 if number >= 0: print("Factorial of", number, "is", factorial(number)) else: print("Enter a positive integer")
This script sets a number
5
then checks if it's non-negative before calling thefactorial
function. The result,120
, demonstrates the correct calculation of 5 factorial.
Recursion and Call Stack Analysis
To fully appreciate recursion, consider how Python manages the call stack during execution:
- On invoking
factorial(5)
, the function doesn't immediately calculate the product but instead calls itself withfactorial(4)
. - This process repeats until
factorial(0)
returns1
. - Python then unwinds the recursion stack, returning the product at each level:
factorial(1)
returns1 * 1 = 1
factorial(2)
returns2 * 1 = 2
- Continuing similarly,
factorial(5)
returns120
Conclusion
Using recursion to calculate the factorial of a number leverages the natural structure of the factorial itself. By implementing the factorial
function recursively, you handle this computation elegantly and efficiently in Python. Remember that recursion can lead to a deep call stack, especially with large numbers, so consider alternatives like iterative approaches or built-in functions for factorial in practical applications. By mastering recursive implementations, you enhance problem-solving skills and deepen your understanding of Python's capabilities.
No comments yet.