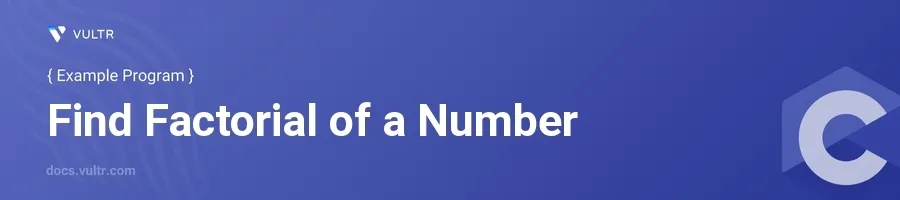
Introduction
The factorial of a number refers to the product of all positive integers less than or equal to that number. For instance, the factorial of 5 is calculated as 5 x 4 x 3 x 2 x 1
, which equals 120. Factorials are commonly used in statistics, combinatorics, and algebra to solve various permutations and combinations problems.
In this article, you will learn how to implement a C program to find the factorial of a given number. Explore both iterative and recursive methods, understand the logic behind each, and review full examples to solidify your understanding of this fundamental concept.
Iterative Method for Factorial Calculation
The iterative approach involves using a loop to multiply numbers repeatedly. Here’s how to implement this method:
Write an Iterative Function to Calculate Factorial
Start by including the standard I/O library.
Declare and define the factorial function using a loop.
Include a main function to take user input and display the result.
c#include <stdio.h> long factorial(int n) { long fact = 1; for (int i = 1; i <= n; i++) { fact *= i; } return fact; } int main() { int number; printf("Enter a positive integer: "); scanf("%d", &number); if (number < 0) printf("Factorial of a negative number doesn't exist.\n"); else printf("Factorial of %d = %ld\n", number, factorial(number)); return 0; }
This program defines a function called factorial
which computes the factorial of a number iteratively. If the user enters a positive number, it calculates and displays the factorial using the factorial function.
Recursive Method for Factorial Calculation
The recursive method calls itself to perform the factorial calculation. This can be an elegant and intuitive approach to solving factorial problems.
Write a Recursive Function to Calculate Factorial
Start by including the standard I/O library.
Declare and define the factorial function which calls itself.
Include a main function to take user input and display the result.
c#include <stdio.h> long factorial(int n) { if (n == 0) // Base case return 1; else return n * factorial(n - 1); // Recursive call } int main() { int number; printf("Enter a positive integer: "); scanf("%d", &number); if (number < 0) printf("Factorial of a negative number doesn't exist.\n"); else printf("Factorial of %d = %ld\n", number, factorial(number)); return 0; }
This version of the program uses recursion to compute the factorial. The factorial
function calls itself with a decrementing value of n
until n
equals zero, which is the base case returning 1.
Conclusion
Factorials can be computed either iteratively or recursively in C programming. While the iterative method uses a simple loop, the recursive method calls itself to reduce the number down to the base case. Both methods are effective for calculating factorials and can be chosen based on the specific requirements of the problem or personal preference. By understanding and implementing these methods, you enhance your problem-solving capabilities in C and gain deeper insights into critical algorithm design concepts.
No comments yet.