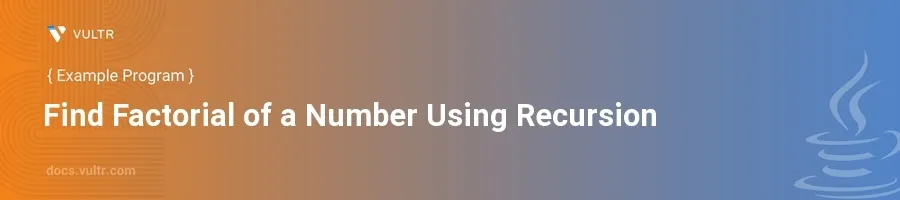
Introduction
Factorial computation, especially using recursion, is a classic example in programming that demonstrates how a problem can be broken down into simpler, repeatable tasks. In mathematics, the factorial of a non-negative integer n is the product of all positive integers less than or equal to n. Recursion is a powerful technique where a method calls itself to solve its sub-problems.
In this article, you will learn how to implement a recursive method to find the factorial of a number in Java. You'll explore a practical coding example, understand the recursive approach, and examine some typical scenarios where you might need to modify the basic implementation to handle larger numbers.
Implementing Recursion to Find Factorials in Java
Basic Recursive Factorial Function
To start, let's write a simple recursive function that computes the factorial of a given number. Follow these steps:
Define a method
factorial
that takes an integern
as its parameter. This integer represents the number you want to find the factorial of.Implement the recursive logic within this method.
- If
n
equals 0, return 1 because the factorial of 0 is 1 by definition. - For any other value of
n
, returnn
multiplied by the factorial ofn-1
.
javapublic class Factorial { public static int factorial(int n) { if (n == 0) { return 1; // Base case: factorial of 0 is 1 } else { return n * factorial(n-1); // Recursive case } } public static void main(String[] args) { int number = 5; // Change this value to compute different factorials System.out.println("Factorial of " + number + " is: " + factorial(number)); } }
In the above Java code, the
factorial
method calls itself withn-1
, thus reducing the problem size with each recursive call until it reaches the base case.- If
Handling Large Factorials with long
Factorials grow rapidly, even for relatively small values of n
. The int
data type may not be sufficient to hold these large values. Using a long
instead of an int
allows for much larger numbers.
Modify the
factorial
method to accept and return along
type.javapublic class Factorial { public static long factorial(long n) { if (n == 0) { return 1; // Base case: factorial of 0 is 1 } else { return n * factorial(n-1); // Recursive case } } public static void main(String[] args) { long number = 20; // Larger number for factorial calculation System.out.println("Factorial of " + number + " is: " + factorial(number)); } }
This modification ensures that the method can handle and return results for larger numbers, up to the limit of the
long
data type.
Conclusion
Understanding and implementing recursion for calculating factorials in Java provides a solid foundation in both recursive thinking and handling potentially large computations in your programming practice. This basic but powerful approach can be enhanced further for optimization or to handle specific edge cases, like negative inputs or extremely large numbers. With this foundation, apply recursion in various other computing problems to simplify solutions and manage complex tasks efficiently.
No comments yet.