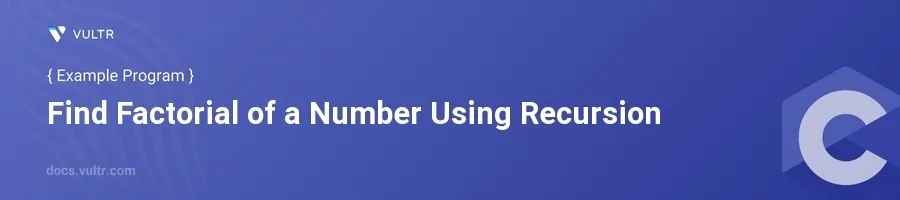
Introduction
Calculating the factorial of a number is a common problem in programming that can be solved using various methods, including recursion. Recursion is a technique where a function calls itself repeatedly until it reaches a base case. In the context of calculating factorials, recursion simplifies the implementation by reducing a factorial problem into simpler, smaller sub-problems.
In this article, you will learn how to implement a recursive function in C to find the factorial of a number. This includes setting up your function, understanding recursion flow, and testing the function with various examples to ensure it works as expected.
Understanding Recursion for Factorial Calculation
Recursion works by breaking down a large problem (like factorial calculation) into smaller problems of the same type. A factorial, represented as n!
, is the product of all positive integers up to n
. It can be defined recursively as follows:
- If
n
is 0, thenn!
is 1 (base case). - Otherwise,
n!
isn
times the factorial ofn-1
(recursive case).
Setting Up the Recursive Function
Begin by including the standard I/O library to handle input and output operations.
Define the recursive function to compute factorial.
c#include <stdio.h> long factorial(int n) { if (n == 0) // Base case return 1; else // Recursive case return n * factorial(n - 1); }
This function returns a
long
value to handle large results. It uses a simple if-else statement to navigate between the base case and the recursive case.
Main Function to Drive the Program
Write a main function which prompts the user to enter a number, calls the factorial function, and displays the result.
cint main() { int num; printf("Enter a number: "); scanf("%d", &num); // Checking if the user enters a negative number if (num < 0) printf("Factorial of a negative number doesn't exist.\n"); else printf("Factorial of %d is %ld\n", num, factorial(num)); return 0; }
This main function handles user input and calls the
factorial
function. It also checks if the input is negative, as factorials of negative numbers are not defined.
Testing the Program
It is essential to test the program with various inputs to ensure it functions correctly:
- Test with Zero: Input
0
to check the base case. - Test with a Small Positive Number: Try numbers like
5
or6
to see the behavior with typical inputs. - Test with Larger Numbers: Use numbers like
15
to see how the program handles more substantial inputs. - Negative Number Input: This tests the program's error handling capabilities.
Expected Outputs for Different Tests
- For
0
, the output should be1
. - For
5
, the output should be120
. - For a negative number, it should notify that factorial doesn't exist.
Conclusion
By implementing the factorial function using recursion in C, you leverage a clean and efficient method that breaks down complex calculations into simpler sub-problems. This recursive approach not only makes your code easier to understand and maintain but also helps in deepening your understanding of how recursion works. Whether it's for educational purposes, technical interviews, or practical application, mastering recursive functions is a valuable skill in any programmer's toolkit.
No comments yet.