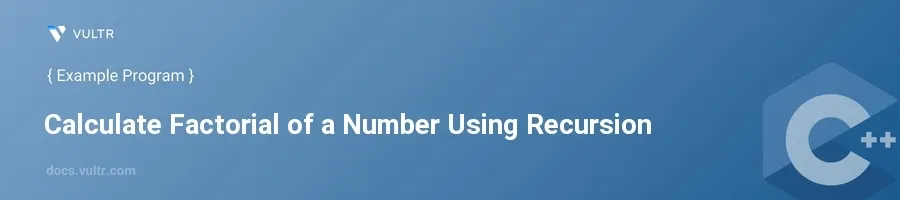
Introduction
In C++, recursion is a powerful method for solving problems by having a function call itself. A classic example of a recursive function is one that computes factorials, the product of all positive integers up to a given number. Factorials are not only fundamental in mathematics but also widely used in statistics, probability, and algorithm analysis.
In this article, you will learn how to write a C++ program to calculate the factorial of a number using recursion. You'll explore how to implement this recursive function and see how it behaves with various input values through multiple examples.
Understanding Factorials and Recursion
Before diving into coding, it's crucial to grasp the concept of recursion and how it applies to calculating factorials.
What is a Factorial?
- The factorial of a non-negative integer (n), denoted as (n!), is the product of all positive integers less than or equal to (n).
- Specifically, (0! = 1) by definition, and for any positive integer (n), (n! = n \times (n-1)!).
How Recursion Works
- A recursive function is a function that calls itself to solve smaller instances of the same problem.
- In the case of calculating a factorial, the recursion repeatedly breaks down the problem into smaller sub-problems by utilizing the relationship (n! = n \times (n-1)!).
- The recursion halts when it reaches the base case, which is typically when (n) equals (0) or (1), as (0! = 1) and (1! = 1).
Implementing the Recursive Function
To calculate the factorial using recursion, define a function that invokes itself until it reaches the base case. Here’s how to implement this.
Define the Recursive Function
Create a function named
factorial
that takes an integer as its parameter.Check if the number is (0) or (1), in which case the function should return (1).
For numbers greater than (1), the function should return the product of the number and the factorial of the number minus one.
cpp#include <iostream> int factorial(int n) { if (n == 0 || n == 1) { // Base case return 1; } else { return n * factorial(n - 1); // Recursive case } }
This function checks if (n) is (0) or (1) and returns (1). Otherwise, it returns (n) times the factorial of (n-1).
Call the Function in main()
In the
main()
function, prompt the user to input a number.Read this number and call the
factorial
function with it.Display the factorial of the given number.
cppint main() { int number; std::cout << "Enter a number: "; std::cin >> number; std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl; return 0; }
This snippet handles user input and outputs the factorial of the input number using the
factorial
function defined earlier.
Examples and Testing
Testing with Different Inputs
- Test with (0) to ensure the base case is correctly handled.
- Try positive numbers such as (5) to validate the recursive calculation.
- Test with (10) or higher to see how the function performs with larger numbers.
For each test case, pay attention to the performance and accuracy of the results to guarantee the recursive function works reliably across a range of inputs.
Conclusion
Utilizing recursion in C++ to calculate factorials exemplifies how simple and elegant solutions can solve seemingly complex problems. The recursive function approach not only makes the code neat and legible but also demonstrates a fundamental programming technique. Expand this knowledge by exploring other problems suited for recursive solutions, like computing Fibonacci series or solving puzzles. By mastering recursion, you enrich your problem-solving toolkit as a programmer, making you better equipped to tackle more challenging problems.
No comments yet.