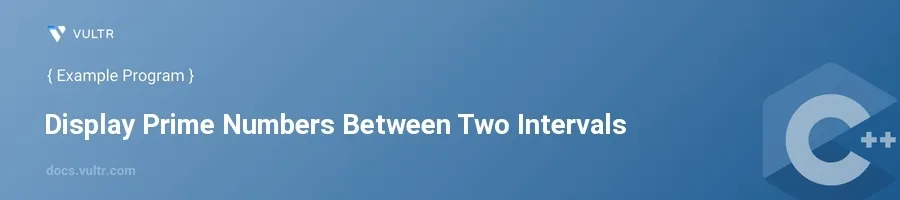
Introduction
Prime numbers are natural numbers greater than 1 that have no positive divisors other than 1 and themselves. Discovering prime numbers within specific intervals is a common exercise in programming, especially when learning a language like C++. This task not only helps in understanding loops and conditions but also in grasping how to effectively manage computational resources in scenarios where efficiency is crucial.
In this article, you will learn how to create a C++ program that identifies and displays all the prime numbers between two intervals specified by the user. Explore the methodologies for efficiently checking prime numbers and how to implement these methods using real C++ examples.
Understanding Prime Number Calculation
Before diving into the program examples, it's essential to understand how prime numbers are determined programmatically.
Basic Method of Prime Checking
Iterate through numbers from 2 to the square root of the target number.
For each number, check if the target number is divisible by it without leaving a remainder.
If it is divisible, the number is not prime.
c++bool isPrime(int num) { if (num <= 1) return false; for (int i = 2; i * i <= num; i++) { if (num % i == 0) return false; } return true; }
This function
isPrime
checks if a single number is prime. It returnsfalse
immediately if a divisor is found, indicating the number is not prime.
Optimization Considerations
- Skip even numbers (except 2) as they are divisible by 2.
- Only numbers greater than 1 can be checked for primality.
Implementing the Prime Number Display
Setting Up the Program
Include necessary headers.
Set up main function to handle user input for intervals.
c++#include <iostream> using namespace std; int main() { int lower, upper; cout << "Enter two intervals: "; cin >> lower >> upper; }
Adding the Logic to Find Primality Between Intervals
Using the
isPrime
function within a loop that goes fromlower
toupper
.c++for (int i = lower; i <= upper; i++) { if (isPrime(i)) { cout << i << " "; } } cout << endl;
This loop checks each number in the specified interval for primality. If a number is prime, it prints the number.
Full Program Example
Bringing together the functions and user interaction into one cohesive program.
c++#include <iostream> using namespace std; bool isPrime(int num) { if (num <= 1) return false; for (int i = 2; i * i <= num; i++) { if (num % i == 0) return false; } return true; } int main() { int lower, upper; cout << "Enter two intervals: "; cin >> lower >> upper; cout << "Prime numbers between " << lower << " and " << upper << ":" << endl; for (int i = lower; i <= upper; i++) { if (isPrime(i)) { cout << i << " "; } } cout << endl; return 0; }
This complete C++ program takes two numbers as input and displays all prime numbers that fall within the specified interval.
Conclusion
Exploring the method of finding prime numbers in a C++ program familiarizes you with fundamental programming concepts like loops, conditionals, and efficiency optimization strategies. By using simple yet effective functions and structures, you develop your coding skills for more complex tasks. Continue tweaking and expanding this program for other mathematical algorithms or computational tasks, and always aim to optimize for the best performance.
No comments yet.