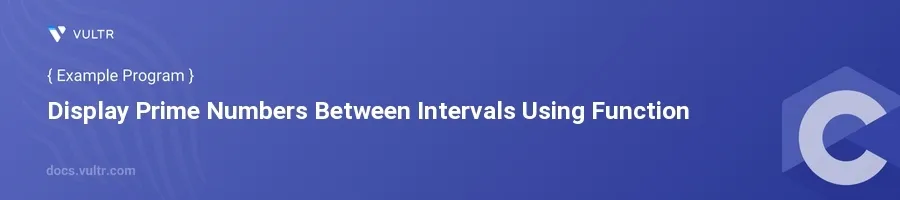
Introduction
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In C programming, there are various methods to check for prime numbers and display them over a range. Understanding how to implement this can enhance your control over loop structures and functions in C.
In this article, you will learn how to create a C program that utilizes functions to display prime numbers between two intervals. Explore how to implement this using functional decomposition, making your code modular and more maintainable.
Prime Number Checking Function
Create the Prime Checking Function
Define a function that takes an integer as an argument and returns a boolean value indicating whether it is a prime number.
Use a loop to check for factors from
2
to the square root of the number, returningfalse
if any factors are found.c#include <stdbool.h> #include <math.h> bool isPrime(int num) { if (num <= 1) return false; for (int i = 2; i <= sqrt(num); i++) { if (num % i == 0) return false; } return true; }
This function checks if
num
is divisible by any number from2
to its square root. If it finds a divisor, it returnsfalse
, indicating the number is not prime. If no divisors are found, it returnstrue
.
Main Function to Display Primes
Read two integers from the user, representing the start and end of the interval.
Iterate over this range, calling
isPrime()
for each number.Print the number if it is prime.
c#include <stdio.h> int main() { int start, end; printf("Enter two numbers(intervals): "); scanf("%d %d", &start, &end); printf("Prime numbers between %d and %d are: ", start, end); for (int num = start; num <= end; num++) { if (isPrime(num)) { printf("%d ", num); } } printf("\n"); return 0; }
In the
main()
function, primes between the user-specified start and end are displayed. It repeatedly callsisPrime()
to determine if a number within the interval is prime and prints it if true.
Conclusion
By leveraging the power of functions in C, you've simplified the task of finding and printing prime numbers between given intervals. This modular approach not only makes the code cleaner but also enhances its reusability. Employ this strategy in your projects to keep your C programs organized and maintainable. Remember, functions are a fundamental tool in the C programmer's toolkit, aiding in the encapsulation and separation of concerns in complex projects.
No comments yet.