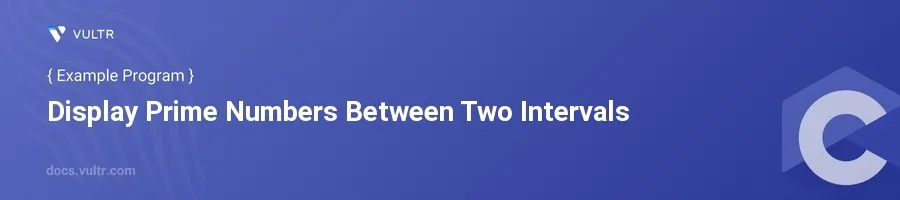
Introduction
A prime number is defined as a number greater than 1, having no positive divisors other than 1 and itself. In C programming, creating a program to find prime numbers between two intervals can be an excellent exercise to understand loops and conditional statements.
In this article, you will learn how to develop a C program that efficiently displays all the prime numbers between two provided intervals. You will explore different examples and see how simple alterations in the approach can affect performance and readability.
Identifying Prime Numbers in C
Basic Prime Checking Function
Create a function to check whether a number is prime. This function returns
1
if the number is prime and0
otherwise.c#include <stdio.h> int isPrime(int num) { if (num <= 1) return 0; for (int i = 2; i * i <= num; i++) { if (num % i == 0) return 0; } return 1; }
This function initially checks if the number is less than or equal to 1, as these are not prime. It then checks divisibility from
2
to the square root of the number, returning0
if any divisor is found.
Main Function to Display Primes Between Intervals
Implement the main function which prompts the user for two intervals and displays prime numbers between them.
cint main() { int low, high; printf("Enter two numbers(intervals): "); scanf("%d %d", &low, &high); printf("Prime numbers between %d and %d are: ", low, high); for (int i = low; i <= high; i++) { if (isPrime(i)) { printf("%d ", i); } } return 0; }
Here, the program reads two numbers defining the intervals. It then iterates through these numbers, using the
isPrime
function to check for prime numbers, and prints each prime found.
Performance Considerations
While the provided implementation works for small intervals, performance may degrade with larger ranges due to the increasing number of checks. Here are some suggestions to improve the program:
- Use a sieve algorithm like the Sieve of Eratosthenes for finding primes in a large range more efficiently.
- Avoid checking even numbers (except for 2) as they are not prime, which can reduce the number of iterations by half.
Conclusion
The C program to display prime numbers between two intervals serves as a practical application of basic programming constructs like loops and functions in C. By testing and tweaking the code, adapt it to handle larger intervals more efficiently or to refine its structure for better understanding and performance. Understanding these principles solidifies foundational programming skills and prepares for more complex problem-solving scenarios.
No comments yet.