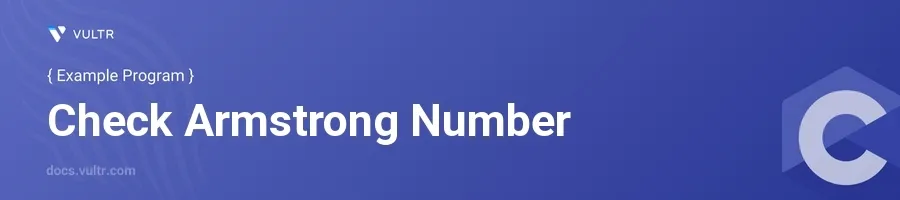
Introduction
An Armstrong number (also known as a narcissistic number) is a number that is equal to the sum of its own digits raised to the power of the number of digits. For example, 153 is an Armstrong number because (1^3 + 5^3 + 3^3 = 153). Understanding how to check for Armstrong numbers in C programming is an interesting challenge that sharpens logic and mastery over loops and conditionals.
In this article, you will learn how to create a C program to determine if a number is an Armstrong number. You will explore examples that cover both basic and advanced scenarios, ensuring a solid grasp of implementing this verification method in the C programming language.
Basic Armstrong Number Check
Determine if a Single Number is an Armstrong Number
Read a number from the user.
Calculate the number of digits in the number.
Sum the cubes (or n-th power) of its digits.
Compare the sum with the original number.
c#include <stdio.h> int main() { int num, original, remainder, sum = 0; int n = 0; // Number of digits printf("Enter an integer: "); scanf("%d", &num); original = num; // Calculate number of digits while (original != 0) { original /= 10; ++n; } original = num; // Sum the nth powers of its digits while (original != 0) { remainder = original % 10; int power = 1; for (int i = 0; i < n; i++) { power *= remainder; } sum += power; original /= 10; } if (sum == num) printf("%d is an Armstrong number.\n", num); else printf("%d is not an Armstrong number.\n", num); return 0; }
This code snippet starts by calculating the number of digits (
n
) in a given number (num
). It then computes the sum of each digit raised to the power ofn
and compares the sum to the original number to determine if it’s an Armstrong number.
Advanced Armstrong Number Check
Validate a Range of Numbers
Iterate over a range of numbers.
Implement the armstrong check for each number in the range.
Display the results accordingly.
c#include <stdio.h> int isArmstrong(int num); int main() { int lower, upper; printf("Enter lower bound: "); scanf("%d", &lower); printf("Enter upper bound: "); scanf("%d", &upper); printf("Armstrong numbers between %d and %d are: ", lower, upper); for (int i = lower; i <= upper; i++) { if (isArmstrong(i)) { printf("%d ", i); } } printf("\n"); return 0; } int isArmstrong(int num) { int original = num, remainder, sum = 0; int n = 0; while (original != 0) { original /= 10; ++n; } original = num; while (original != 0) { remainder = original % 10; int power = 1; for (int i = 0; i < n; i++) { power *= remainder; } sum += power; original /= 10; } return sum == num; }
This enhanced version defines a function
isArmstrong()
to check if a single number is an Armstrong number. It then iterates over a user-defined range, checking each number using the previously defined function and prints those that are Armstrong numbers.
Conclusion
The process of checking Armstrong numbers in C involves understanding loops, conditional statements, and basic mathematical operations. By implementing and iterating on the examples provided, you gain deeper insights into both C syntax and more complex programming concepts like function encapsulation and reusability. Use these techniques to further enrich your understanding of how numerical problems can be tackled effectively in C programming.
No comments yet.