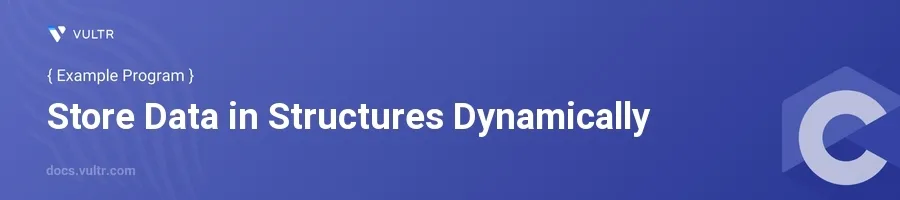
Introduction
Dynamic memory allocation in C programming plays a crucial role, especially when the memory requirement isn't known beforehand and can vary. Using structures to store data dynamically combines structured data handling with memory efficiency. This approach is particularly useful in scenarios like handling records in a database system, where each record may contain various fields but the total number of records is not fixed.
In this article, you will learn how to dynamically allocate memory for structures in C, effectively manage memory, and utilize these structures to store data dynamically. Several practical examples will demonstrate how to implement these techniques efficiently in real-world scenarios.
Allocating Memory Dynamically for Structures
Define a Structure
Define the structure with the relevant fields.
ctypedef struct { int id; char name[100]; float salary; } Employee;
This code defines an
Employee
structure with anint
for ID, a character array for the name, and afloat
for the salary.
Allocating Memory Using malloc()
Use the
malloc()
function to dynamically allocate memory for the structure.cEmployee* create_employee(int id, char* name, float salary) { Employee* new_employee = (Employee*)malloc(sizeof(Employee)); if (new_employee == NULL) { fprintf(stderr, "Memory allocation failed\n"); return NULL; } new_employee->id = id; strcpy(new_employee->name, name); new_employee->salary = salary; return new_employee; }
The
malloc()
function allocates memory the size of theEmployee
structure. This function returns a pointer to the allocated memory block, which is then used to access the structure fields.
Using Structures in Programs
Store Multiple Records Dynamically
Create an array of pointers to handle multiple
Employee
records dynamically.cint main() { int num_employees = 5; Employee* employees[num_employees]; employees[0] = create_employee(1, "Alice", 50000); employees[1] = create_employee(2, "Bob", 60000); employees[2] = create_employee(3, "Charlie", 55000); employees[3] = create_employee(4, "David", 50000); employees[4] = create_employee(5, "Eve", 65000); for (int i = 0; i < num_employees; i++) { if (employees[i] != NULL) { printf("ID: %d, Name: %s, Salary: %.2f\n", employees[i]->id, employees[i]->name, employees[i]->salary); } } // Cleanup for (int i = 0; i < num_employees; i++) { free(employees[i]); } return 0; }
This code fragment initializes an array of
Employee
pointers and assigns memory to each using thecreate_employee
function. Finally, it frees the allocated memory to avoid memory leaks.
Conclusion
Using dynamic memory allocation for structures in C allows for efficient data management where the amount of data is not known beforehand. By combining structured data access with dynamic memory management, programs can handle variable data sizes elegantly and efficiently. The examples provided illustrate how to create, manage, and free dynamic structures, serving as a groundwork for more complex data management tasks in your C programming projects.
No comments yet.