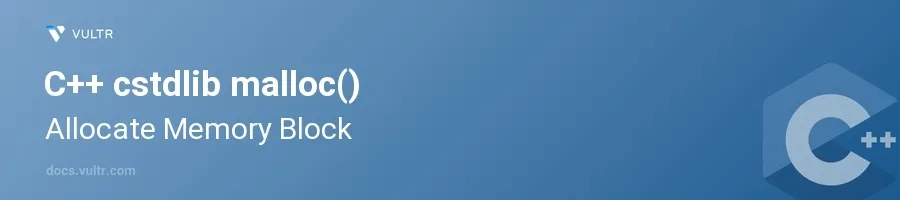
Introduction
malloc()
is a fundamental memory allocation function in the C and C++ programming languages, defined in the C standard library (cstdlib
). This function allocates a specific amount of memory during runtime, which is essential for dynamic memory management in applications dealing with variable-sized data structures like arrays, linked lists, or binary trees.
In this article, you will learn how to effectively use malloc()
for dynamic memory allocation in C++. You’ll discover various scenarios where malloc()
can be applied, how to check for successful allocation, and how to properly deal with memory management to avoid leaks and other issues.
Using malloc()
in C++
Basic Memory Allocation
Include the C standard library header.
Allocate memory using
malloc()
.Verify the success of the memory allocation.
Demonstrate the use of allocated memory.
Free the allocated memory.
cpp#include <cstdlib> #include <iostream> int main() { int *ptr = (int*)malloc(sizeof(int)); if (ptr == nullptr) { std::cerr << "Memory allocation failed." << std::endl; return 1; } *ptr = 5; std::cout << "Value: " << *ptr << std::endl; free(ptr); return 0; }
In this example, memory for one integer is allocated. After checking that
malloc()
didn't returnnullptr
(which would indicate failure), the program assigns a value to the allocated memory, prints it, and then properly frees the memory.
Allocating an Array of Elements
Determine the number of elements to allocate.
Use
malloc()
to allocate memory for the array.Initialize the elements of the array.
Free the allocated memory.
cpp#include <cstdlib> #include <iostream> int main() { size_t num_elements = 10; int *array = (int*)malloc(num_elements * sizeof(int)); if (array == nullptr) { std::cerr << "Memory allocation for array failed." << std::endl; return 1; } for (size_t i = 0; i < num_elements; ++i) { array[i] = i * i; std::cout << "array[" << i << "] = " << array[i] << std::endl; } free(array); return 0; }
Here,
malloc()
is used to allocate memory for an array of 10 integers. Each element is initialized to the square of its index. Finally, the array is freed usingfree()
.
Common Pitfalls and Best Practices
Handling Memory Allocation Failures
- Always check if
malloc()
returnsnullptr
as this indicates an allocation failure. - Handle the failure appropriately, usually with a cleanup and an error message.
Avoiding Memory Leaks
- Always pair
malloc()
withfree()
to prevent memory leaks. - Consider using smart pointers in C++ for automatic memory management.
Using malloc()
in Modern C++
- Typically, in modern C++, it's recommended to use C++ memory management techniques such as
new
anddelete
instead ofmalloc()
andfree()
. - For safer and more convenient memory management, consider using smart pointers (
std::unique_ptr
,std::shared_ptr
) which handle automatic deallocation.
Conclusion
While malloc()
serves as a crucial tool for memory allocation in C++, modern C++ practices favor constructors and destructors, along with memory management classes and templates for improved safety and readability. Understanding and using malloc()
still proves very useful, especially when interacting with C code or when low-level memory control is necessary. Practice using malloc()
correctly to master memory management in both C and C++ environments, and remember to transition to modern C++ techniques as you evolve your programming skills.
No comments yet.