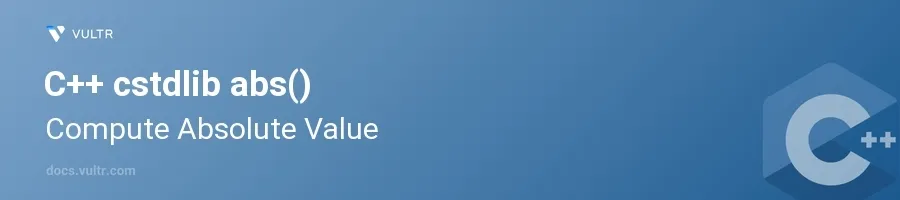
Introduction
The abs()
function in C++, provided by the <cstdlib>
header file, is a straightforward utility used for computing the absolute (non-negative) value of integers. This function is essential in scenarios where you need to ensure that a numerical value is non-negative, such as in mathematical calculations, algorithms that measure distances, or when handling data that requires non-negative integers.
In this article, you will learn how to efficiently utilize the abs()
function in your C++ programs. Explore its implementation and practical applications with concrete examples that demonstrate how to handle different data scenarios using abs()
.
Understanding abs() Function
Basic Usage of abs()
Include the
<cstdlib>
library in your C++ program.Pass an integer value to the
abs()
function.Capture and utilize the returned absolute value.
cpp#include <cstdlib> #include <iostream> int main() { int num = -10; int result = abs(num); std::cout << "The absolute value of " << num << " is " << result << std::endl; }
This code snippet demonstrates using
abs()
to convert a negative integer to a positive one. The program will output "The absolute value of -10 is 10".
Handling Variable Inputs
Work with variables and ensure the function appropriately computes absolute values for both positive and negative integers.
Extend the example to handle user input, reinforcing the dynamic utility of
abs()
.cpp#include <cstdlib> #include <iostream> int main() { int num; std::cout << "Enter a number: "; std::cin >> num; int result = abs(num); std::cout << "The absolute value of " << num << " is " << result << std::endl; }
In this example, the
abs()
function processes user input. Whether the user enters a negative or non-negative integer, the output will be the absolute value of the intput number.
Working with Larger Integers
Using abs() with Long Integers
Recognize the need for
labs()
when dealing with long integers, asabs()
is typically used forint
type.Utilize
labs()
for absolute calculations on long integers.cpp#include <cstdlib> #include <iostream> int main() { long num = -1234567890L; long result = labs(num); std::cout << "The absolute value of " << num << " is " << result << std::endl; }
This code makes use of
labs()
, which functions similarly toabs()
but is designed forlong
integers. The result is the non-negative version of the provided long integer.
Conclusion
The abs()
function from the C++ <cstdlib>
library plays a crucial role in numerous programming tasks, particularly those requiring manipulation and assurance of non-negative numbers. By mastering the use of abs()
and its variations like labs()
, you effectively handle various data types and ensure your calculations are error-free with respect to negative values. Apply these strategies to enhance numerical stability and reliability in your C++ projects.
No comments yet.