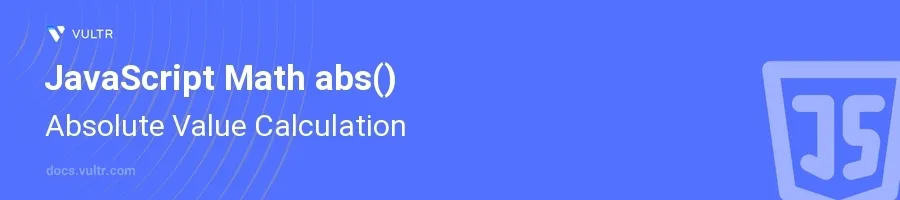
Introduction
The Math.abs()
function in JavaScript is a straightforward yet powerful utility that calculates the absolute value of a given number. Absolute value refers to the non-negative value of a number without regard to its sign. This function is essential in various programming contexts, especially in mathematical computations, where determining the magnitude of a number regardless of its polarity is required.
In this article, you will learn how to effectively utilize the Math.abs()
function across different programming scenarios. Explore its benefits in handling both positive and negative numbers and see how it affects decimal values and special cases.
Basic Usage of Math.abs()
Calculating the Absolute Value
Pass a negative number to
Math.abs()
to observe its conversion to positive.Execute the function with a positive number to see that it remains unchanged.
javascriptconst negativeNum = -10; const positiveNum = 5; const absNegative = Math.abs(negativeNum); const absPositive = Math.abs(positiveNum); console.log(absNegative); // Outputs: 10 console.log(absPositive); // Outputs: 5
This code demonstrates that
Math.abs()
successfully converts a negative number to its positive equivalent while leaving a positive number unchanged.
Handling Decimal Values
Understand that the function works equally well with decimal numbers.
Apply
Math.abs()
to a decimal value.javascriptconst decimalNum = -5.67; const absDecimal = Math.abs(decimalNum); console.log(absDecimal); // Outputs: 5.67
Here,
Math.abs()
effectively returns the positive version of the negative decimal, demonstrating its capability with floating-point numbers.
Dealing with Special Cases
Non-numeric Inputs
Recognize that non-numeric inputs typically return
NaN
.Test
Math.abs()
with a string and other invalid types to see the output.javascriptconst invalidInput = "hello"; const absInvalid = Math.abs(invalidInput); console.log(absInvalid); // Outputs: NaN
This example illustrates that
Math.abs()
will returnNaN
when handed non-numeric input, emphasizing the need for type checking in dynamic languages like JavaScript.
Edge Cases
Explore JavaScript specificities with
Math.abs()
, like handlingInfinity
,null
, and-0
.Observe the behavior of the function with these special values.
javascriptconsole.log(Math.abs(Infinity)); // Outputs: Infinity console.log(Math.abs(-Infinity)); // Outputs: Infinity console.log(Math.abs(null)); // Outputs: 0 console.log(Math.abs(-0)); // Outputs: 0
Math.abs()
returnsInfinity
for bothInfinity
and-Infinity
inputs, turnsnull
into0
, and also converts-0
to0
, showcasing its handling of JavaScript-specific edge cases.
Conclusion
The Math.abs()
function in JavaScript is indispensable for absolute value calculations, offering reliable results across a spectrum of numeric and non-numeric inputs. By integrating Math.abs()
into your JavaScript projects, you can effortlessly handle mathematical operations that require magnitude assessments, ensuring your calculations are both accurate and efficient. Adopt these techniques to bolster your programming toolkit and streamline complex computations in JavaScript environments.
No comments yet.