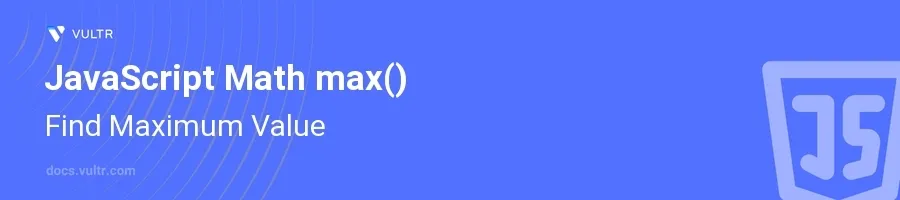
Introduction
The Math.max()
method in JavaScript is a straightforward yet powerful tool used to determine the maximum value among its given arguments. By accepting multiple numbers or an array of numbers (with a spread operator), this method evaluates and returns the largest number in the set, facilitating numerical comparisons directly in your code.
In this article, you will learn how to leverage the Math.max()
method to find the maximum value in various scenarios. Explore how to use this function with different sets of numbers, understand its return behavior in special cases, and see how it compares with alternative approaches.
Using Math.max() with Numbers
Find Maximum among Multiple Numbers
Directly pass numerical values as arguments to
Math.max()
.Print or utilize the result as needed.
javascriptconst maxVal = Math.max(5, 10, 15, 20); console.log(maxVal);
This code evaluates the given numbers and outputs the highest value, which is
20
.
Handling Variable Numbers of Arguments
Use the spread operator to pass an array of numbers to
Math.max()
.javascriptconst numbers = [5, 10, 15, 20]; const maxVal = Math.max(...numbers); console.log(maxVal);
The spread operator (
...
) allows an array to be expanded into individual elements, making it possible to determine the maximum value among the elements in the array,20
.
Special Cases in Using Math.max()
Understanding Return Value for No Arguments
Recognize that calling
Math.max()
without any arguments returns-Infinity
.javascriptconst maxVal = Math.max(); console.log(maxVal);
Since no arguments are provided,
Math.max()
returns-Infinity
. This could be useful in scenarios where you need a conditional minimum benchmark.
Dealing with Non-Number Inputs
Note how
Math.max()
handles non-numeric inputs or mixed types.javascriptconst result = Math.max(10, '20', null); console.log(result);
In this scenario,
Math.max()
coercesnull
to0
and attempts to convert strings to numbers, thus returning20
.
Edge Cases and Comparisons
Comparing with Zeros and Negative Values
Evaluate how
Math.max()
treats0
,-0
, and negative numbers.javascriptconst result = Math.max(-10, 0, -0, 20); console.log(result);
The method identifies
20
as the maximum value. Note that JavaScript treats0
and-0
as equal, and both are considered less than any positive number.
Performance Considerations
Understand that direct comparisons using
Math.max()
are typically more performant and concise than manual iterative approaches.javascriptconst largeNumArray = Array(1000000).fill().map((_, i) => i); console.log(Math.max(...largeNumArray));
This code generates a large array of numbers and efficiently finds the maximum value. The performance of
Math.max()
using the spread operator is usually satisfactory for most practical applications but can depend on the specific JavaScript engine and its optimization strategies.
Conclusion
The Math.max()
method in JavaScript simplifies the task of finding the highest numerical value among given arguments. By understanding how to effectively apply this method in various scenarios, including handling special cases and performance considerations, you enhance your capability to write concise, efficient, and readable JavaScript code. Whether dealing with small sets of numbers, large arrays, or mixed data types, Math.max()
provides a robust solution for numerical comparisons.
No comments yet.