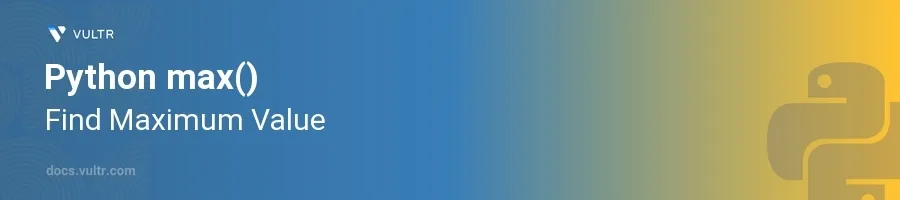
Introduction
The max()
function in Python is a built-in utility that identifies the maximum value among the provided arguments or elements within an iterable. This function is widely utilized across different types of data structures such as lists, tuples, and dictionaries, making it versatile for various programming scenarios where determining the highest value is crucial.
In this article, you will learn how to effectively use the max()
function to find the maximum value in different contexts. Explore how to apply this function to simple and complex data structures, and how to handle cases with custom criteria.
Using max() with Lists
Find the Maximum Number in a List
Create a list of numeric values.
Use the
max()
function to determine the highest number.pythonnumbers = [5, 10, 3, 18, 1] max_value = max(numbers) print(max_value)
This code initializes a list
numbers
and then usesmax()
to find the largest value. The output will be18
, as it is the highest number in the list.
Handling Strings in Lists
Recognize that
max()
can also be used to find the "maximum" element in a list of strings, based on lexicographical order.Prepare a list containing string elements.
Apply the
max()
function to find the string that comes last alphabetically.pythonwords = ["banana", "apple", "cherry"] max_word = max(words) print(max_word)
Here, the
max()
findscherry
as the maximum since it compares string values based on alphabetical order.
Using max() with Dictionaries
Find the Maximum Among Dictionary Values
Understand that by default, applying
max()
to a dictionary evaluates only the keys.Utilize the
values()
method to direct the function to assess the dictionary's values instead.Use
max()
to find the highest value among the dictionary values.pythondata = {'a': 100, 'b': 200, 'c': 50} max_value = max(data.values()) print(max_value)
This example directs
max()
to evaluate the values of the dictionarydata
, resulting in200
being identified as the maximum value.
Compare Items Based on Custom Key Function
Learn that
max()
allows for a custom key function to specify the criteria for comparison.Use a key function to assess elements based on a specific attribute or predefined logic.
Apply
max()
with the key function to identify the desired maximum element.pythonfruits = [{'name': 'apple', 'weight': 0.5}, {'name': 'banana', 'weight': 0.3}, {'name': 'cherry', 'weight': 0.2}] max_fruit = max(fruits, key=lambda fruit: fruit['weight']) print(max_fruit['name'])
Here,
max()
uses a lambda function to compare fruits based on their 'weight' attribute. The output will showapple
as it has the highest weight in the list.
Conclusion
The max()
function in Python provides a straightforward, yet powerful means of finding the maximum value across various data structures. Its flexibility to work with simple lists, complex dictionaries, and custom criteria via key functions makes it an indispensable tool in Python programming. Enhance your code effectiveness by incorporating the max()
function in suitable scenarios to streamline data comparison tasks. By mastering this function, you ensure your code efficiently identifies the maximum values in multiple contexts and data formats.
No comments yet.