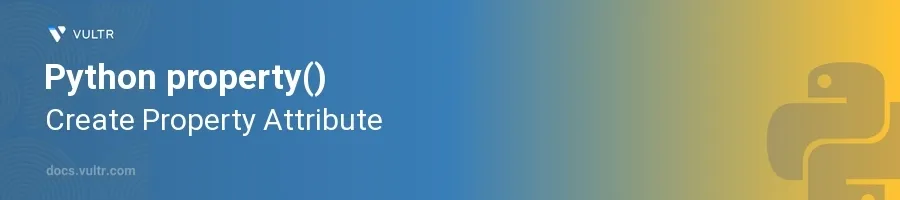
Introduction
The property()
function in Python is a built-in function that provides a neat way of managing instance attributes. It effectively encapsulates attribute access, enabling attribute values to be accessed like simple instance attributes while actually providing methods to get, set, and delete the attribute. This feature is crucial for implementing data encapsulation and adhering to the principle of least privilege in object-oriented programming.
In this article, you will learn how to leverage the property()
function to create and manage property attributes in Python classes. Discover the benefits of using properties for controlled attribute access and how to define clean interfaces to your objects.
Using property() with Python Classes
Creating a Simple Read-only Property
Define a class with an internal attribute.
Use the
property()
function to create a read-only property.pythonclass Circle: def __init__(self, radius): self._radius = radius @property def radius(self): return self._radius
In this example,
radius
is treated as a read-only property of theCircle
class. Theproperty()
decorator makesradius
accessible, but modification is restricted, makingradius
immutable from outside class methods.
Adding a Setter to the Property
Continue with the previous class structure.
Add a setter method to allow property modification.
pythonclass Circle: def __init__(self, radius): self._radius = radius @property def radius(self): return self._radius @radius.setter def radius(self, value): if value >= 0: self._radius = value else: raise ValueError("Radius cannot be negative")
This code adds a
setter
for theradius
property, which allows the modification of_radius
if the new value is non-negative. The setter ensures that the radius remains a valid value, enforcing a simple data validation directly through the property interface.
Implementing a Deleter for Property
Expand the existing class to include a deleter.
Use the deleter to handle property deletion appropriately.
pythonclass Circle: def __init__(self, radius): self._radius = radius @property def radius(self): return self._radius @radius.setter def radius(self, value): if value >= 0: self._radius = value else: raise ValueError("Radius cannot be negative") @radius.deleter def radius(self): print("Deleting radius") del self._radius
The
deleter
defines what happens when the property is deleted. In this case, deletingradius
from an instance ofCircle
will remove the_radius
attribute and print a message. This level of control over how properties are handled is one of the primary benefits of usingproperty()
.
Conclusion
The property()
function in Python is powerful for creating properties that behave like instance variables but include management logic for setting, getting, and deleting their values. By utilizing property()
, you enhance the encapsulation and abstraction capabilities of Python classes, ensuring better management of state and adherence to data protection principles. Whether you need read-only attributes, write-protected data, or other controlled access patterns, the techniques discussed here enable you to maintain clean and efficient class interfaces.
No comments yet.