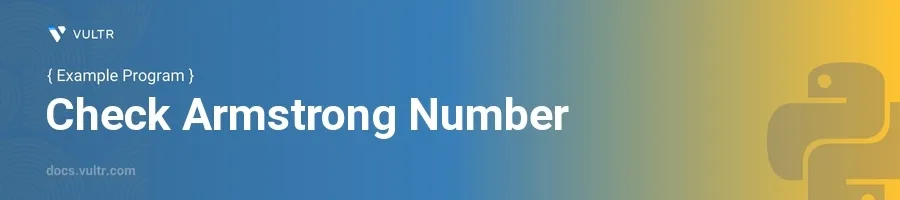
Introduction
An Armstrong number (also known as a narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because (1^3 + 5^3 + 3^3 = 153). This concept is not only intriguing in number theory but also a popular problem in computer programming interviews and examinations.
In this article, you will learn how to determine if a number is an Armstrong number using Python. Through hands-on examples, explore effective techniques to implement this check programmatically, aiding in better understanding and grasp of Python's computational abilities.
Identifying Armstrong Numbers in Python
Single Example
Check if a specific number is an Armstrong number by following these steps:
Convert the number to a string to easily iterate over each digit.
Calculate the total number of digits (length of the string).
Sum the cubes (or appropriate power) of each digit.
Compare the sum to the original number to ascertain if it's an Armstrong number.
pythonnum = 153 num_str = str(num) num_len = len(num_str) armstrong_sum = sum([int(digit) ** num_len for digit in num_str]) if armstrong_sum == num: print(f"{num} is an Armstrong number.") else: print(f"{num} is not an Armstrong number.")
This code converts the number
153
to a string, iterates over each character in the string, raises it to the power of the number of digits, and sums the results. It then checks whether the sum equals the original number.
Checking a Range of Numbers
To find all Armstrong numbers within a range, employ the following approach:
Loop through each number in the specified range.
For each number, repeat the process used in the single example to determine if it's an Armstrong number.
Print the number if it meets the Armstrong condition.
pythonlower_limit = 100 upper_limit = 1000 for num in range(lower_limit, upper_limit): num_str = str(num) num_len = len(num_str) armstrong_sum = sum(int(digit) ** num_len for digit in num_str) if armstrong_sum == num: print(f"{num} is an Armstrong number.")
The code iterates from
100
to1000
, checks each number, and prints it if it's an Armstrong number. It reuses the conversion, power, and summing logic described previously.
Conclusion
Checking whether a number is an Armstrong number in Python demonstrates the application of loops, conditional statements, and list comprehensions. Using these basic constructs, you can solve common mathematical problems effectively. The techniques discussed serve as a solid foundation for tackling similar problems, enhancing your problem-solving and Python programming skills. Apply these methods in your projects or while preparing for coding interviews.
No comments yet.