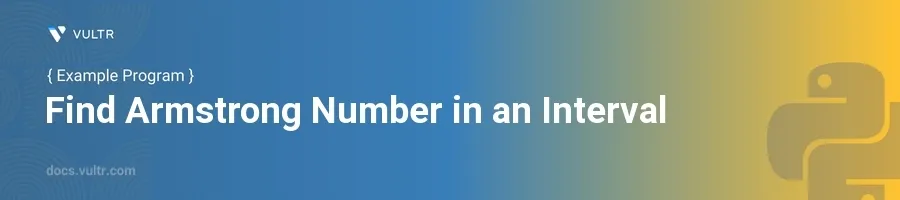
Introduction
An Armstrong number (or Narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because (1^3 + 5^3 + 3^3 = 153). These numbers are intriguing due to their self-representative nature and are often a popular subject in programming exercises to practice loops and conditionals.
In this article, you will learn how to write a Python program to find all Armstrong numbers in a given interval. This task will help you understand how to implement loops, conditional statements, and basic arithmetic operations in Python.
Finding Armstrong Numbers within an Interval
Determine if a Number is an Armstrong Number
Define a function to check if a number is an Armstrong number. The function takes a single integer as an argument.
Convert the number to a string to easily iterate over each digit.
Calculate the total number of digits in the number.
Sum the cubes (or powers corresponding to the digit length) of each digit.
Compare the sum to the original number and return
True
if they match, indicating it is an Armstrong number.pythondef is_armstrong(num): str_num = str(num) power = len(str_num) sum_of_powers = sum(int(digit) ** power for digit in str_num) return sum_of_powers == num
This function handles the task of calculating if a single number is an Armstrong number based on the definition. The digits are raised to the power of the total number of digits and then summed to see if they equal the original number.
Loop through an Interval to Find Armstrong Numbers
Accept two integers as the start and end of the interval.
Iterate through each number in this range.
Use the previously defined
is_armstrong()
function to check each number.Append the number to a list if it is an Armstrong number.
Print the results after completing the loop through the range.
pythondef find_armstrong_numbers(start, end): armstrong_list = [] for num in range(start, end + 1): if is_armstrong(num): armstrong_list.append(num) return armstrong_list
This function performs a loop from
start
toend
, checking each number to see if it's an Armstrong number via theis_armstrong()
function. It collects all Armstrong numbers found within this interval.
Example Usage
To see the functions in action, define a range and find Armstrong numbers within it.
start_interval = 100
end_interval = 2000
armstrong_numbers = find_armstrong_numbers(start_interval, end_interval)
print("Armstrong numbers in interval:", armstrong_numbers)
This script sets an interval from 100 to 2000 and prints the Armstrong numbers found within this range. Adjust the interval values to explore other ranges.
Conclusion
Finding Armstrong numbers within a specified interval in Python offers a solid exercise in understanding loops, conditionals, and mathematical operations. Utilize the steps outlined to create efficient, readable Python scripts that accurately identify these unique numbers. This exercise not only helps in strengthening programming skills but also aids in grasping the practical applications of elementary mathematical concepts in computer science.
No comments yet.