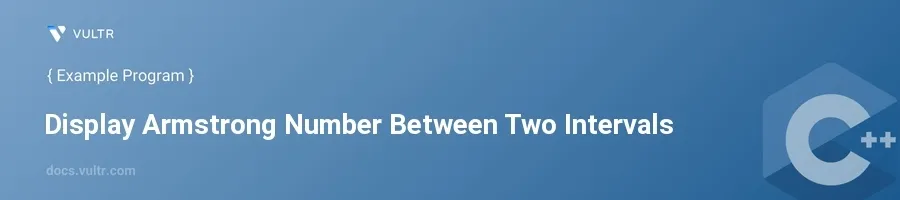
Introduction
An Armstrong number, also known as a narcissistic number, is a number that is equal to the sum of its own digits each raised to the power of the number of digits. This interesting property makes Armstrong numbers a popular subject in programming exercises, especially in educational settings to teach loops and conditionals.
In this article, you will learn how to create a C++ program that displays all Armstrong numbers between two intervals. Explore how to implement the logic to check for Armstrong numbers, and how to iterate through a range of values effectively using C++.
Understanding Armstrong Numbers
Before diving into the code, it's essential to understand the concept of an Armstrong number:
- An Armstrong number for a three-digit number would be: ( ABC = A^3 + B^3 + C^3 )
- For a four-digit number, it would be: ( ABCD = A^4 + B^4 + C^4 + D^4 )
The challenge lies not only in checking if a number fits this criteria but also in dynamically adjusting the power based on the number of digits.
Writing the C++ Program
Setup Your Development Environment
- Ensure you have a C++ compiler installed, such as GCC or Clang.
- Open a text editor or an IDE like Visual Studio Code, which supports C++ programming.
Create the Function to Check Armstrong Number
Start by defining a function that determines if a number is an Armstrong number.
This function should calculate the sum of each digit raised to the power of the total number of digits.
cpp#include <iostream> #include <cmath> bool isArmstrong(int num) { int original = num, sum = 0; int n = 0; while (num != 0) { n++; num /= 10; } num = original; while (num != 0) { int digit = num % 10; sum += pow(digit, n); num /= 10; } return original == sum; }
This function iterates through each digit of the number, calculating the sum of each digit raised to the power of the total number of digits, and checks if it matches the original number.
Create the Main Function
Develop the main segment of the program to accept two numbers that define the range between which you want to find Armstrong numbers.
Use a for loop to iterate through this range and use the
isArmstrong
function to check each number.cppint main() { int low, high; std::cout << "Enter two numbers (low and high): "; std::cin >> low >> high; std::cout << "Armstrong numbers between " << low << " and " << high << " are: "; for(int i = low; i <= high; i++) { if(isArmstrong(i)) { std::cout << i << " "; } } std::cout << std::endl; return 0; }
This code snippet will output all the Armstrong numbers between the provided lower and upper bounds.
Testing Your Program
After compiling the program, run it to verify its functionality. Test it with different intervals to see how it performs and ensure it correctly identifies Armstrong numbers.
Conclusion
By following the steps outlined in this article, you have created a robust C++ program capable of identifying Armstrong numbers within a given range. This task not only reinforces your understanding of basic programming constructs in C++ such as loops and functions but also provides a practical application of mathematical concepts in computer programming. Continue to experiment with this program, perhaps by optimizing it or by modifying it to handle larger numbers or different types of inputs.
No comments yet.