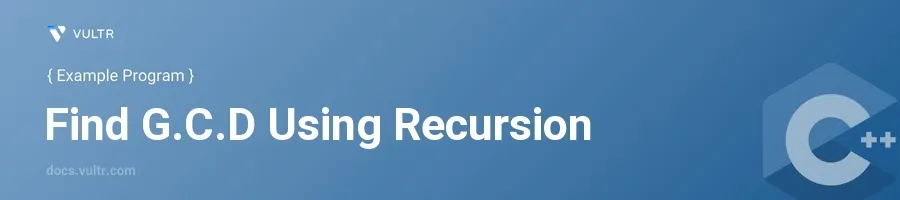
Introduction
The Greatest Common Divisor (G.C.D), also known as the Greatest Common Factor (G.C.F) or Highest Common Factor (H.C.F), is the largest positive integer that divides two or more integers without leaving a remainder. In programming, calculating the G.C.D is a common task that can be efficiently implemented using recursion, especially in C++ due to its performance benefits with recursive functions.
In this article, you will learn how to leverage recursion in C++ to find the G.C.D of two numbers. Demonstrated through clear examples, you will discover how recursive functions streamline code in implementing mathematical algorithms such as the Euclidean algorithm for computing G.C.D.
Implementing G.C.D. Calculation with Recursion
Understanding the Euclidean Algorithm
The Euclidean algorithm is based on the principle that the G.C.D of two numbers also divides their difference. This key insight reduces the problem size at each step, making it particularly suitable for a recursive solution in C++.
Recursive Function for G.C.D
Identify base cases for recursion. In the context of G.C.D calculation:
- If the second number is zero, the G.C.D is the first number.
Formulate the recursive function. If both numbers are non-zero, recursively call the function by passing the smaller number and the remainder of the division of the larger number by the smaller number.
cpp#include <iostream> using namespace std; int gcd(int a, int b) { if (b == 0) return a; return gcd(b, a % b); } int main() { int num1 = 48, num2 = 18; cout << "G.C.D of " << num1 << " and " << num2 << " is: " << gcd(num1, num2) << endl; return 0; }
In this C++ program, the
gcd
function is defined to calculate the G.C.D of two integers,a
andb
. Ifb
is zero, it returnsa
. Otherwise, it calls itself withb
and the remainder ofa
divided byb
. Themain
function initializes two numbers, callsgcd
, and outputs the result.
Analysis of the Recursive Solution
- The function decreases the problem size at every recursive call by using the remainder operation, which ensures that the smaller argument is gradually reduced towards zero.
- One of the major strengths of this approach is its succinctness and clarity, closely mirroring the mathematical definition and process of the Euclidean algorithm.
Advanced Example: Handling Negative Numbers and Zero
Extending Functionality
Adjust the base case to handle negative numbers, by returning the absolute value of the number.
Test the extended gcd function with different pairs including zero and negative values.
cpp#include <iostream> #include <cmath> // Include cmath for using the abs function using namespace std; int gcd(int a, int b) { if (b == 0) return abs(a); return gcd(b, a % b); } int main() { cout << "G.C.D of -36 and 24 is: " << gcd(-36, 24) << endl; cout << "G.C.D of 0 and 45 is: " << gcd(0, 45) << endl; cout << "G.C.D of 0 and 0 is: " << gcd(0, 0) << endl; return 0; }
In these examples, negative numbers are handled by the
abs
function which ensures the return of a non-negative G.C.D. The function is also tested with zero inputs, demonstrating its robustness in different scenarios.
Conclusion
Leveraging recursion in C++ for calculating the G.C.D of two numbers with the Euclidean algorithm is an elegant and efficient approach. As you've seen through the examples, a correct base case and the recursive formulation are crucial to crafting a functional recursive solution. This method not only follows the mathematical logic closely but also optimizes handling cases like negative numbers and zeros. Begin incorporating these recursive strategies in mathematical algorithms to make your C++ programs more efficient and maintainable.
No comments yet.