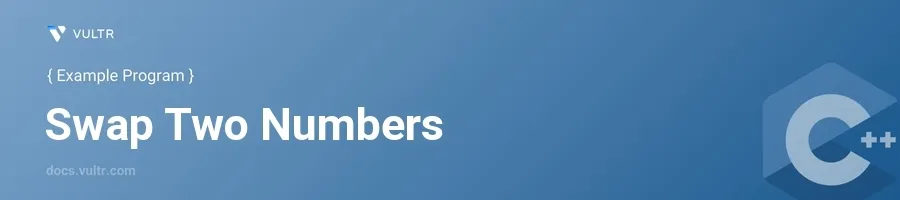
Introduction
Swapping two numbers in C++ programming involves interchanging their values. This is a fundamental operation used in sorting algorithms, memory management, and data manipulation. In C++, swapping can be implemented in several ways, including using temporary variables, arithmetic operations, and the standard library functions. Understanding how to swap values effectively is crucial for optimizing algorithms and solving programming problems efficiently.
In this article, you will learn how to write a C++ program to swap two numbers through various methods.. These will include using a temporary variable, performing the swap without a third variable using arithmetic and XOR operations, and utilizing C++'s built-in functions. Each method will be illustrated with clear examples, ensuring you understand the concepts and can apply them in different scenarios.
Using a Temporary Variable
Swapping two numbers with the help of a temporary storage variable is the most straightforward approach. Follow these steps to implement this method:
Declare three variables: two for the numbers you want to swap and one to temporarily hold the value during the swap.
Assign values to the two main variables.
Perform the swap by using the temporary variable.
cpp#include<iostream> using namespace std; int main() { int a = 5; int b = 10; int temp; // Perform the swap temp = a; a = b; b = temp; cout << "After swap, a = " << a << " and b = " << b << endl; return 0; }
In this example,
temp
is used to temporarily hold the value ofa
. Thena
is set to the value ofb
, and finally,b
is set to the value stored intemp
. The result is that the values ofa
andb
are swapped.
Swap Without a Third Variable
Write a C++ program to swap two numbers without using a third variable by leveraging arithmetic operations like addition and subtraction. This technique allows for efficient value exchange between variables without the need for extra storage. For instance, by adding both numbers and then adjusting each variable accordingly, their values can be swapped seamlessly. This approach not only simplifies the code but also optimizes performance, making it a valuable method in resource-constrained environments.
Using Arithmetic Operations
Swapping two numbers without an extra variable can be achieved using arithmetic operations like addition and subtraction. Note that this approach might result in data overflow if using very large integers or underflow for very small numbers.
Assign values to the two variables you want to swap.
Use addition and subtraction to swap the values without a temporary variable.
cpp#include<iostream> using namespace std; int main() { int a = 5; int b = 10; // Swap without temp using arithmetic a = a + b; // a becomes 15 b = a - b; // b becomes 5 a = a - b; // a becomes 10 cout << "After swap, a = " << a << " and b = " << b << endl; return 0; }
Here, the sum of
a
andb
is stored ina
, thenb
is subtracted from this newa
to get the originalb
value, and finallyb
is subtracted from the newa
to recover the originala
.
Using XOR Bitwise Operation
The XOR bitwise operation can also be used to swap two numbers without a third variable. This method avoids potential overflow issues but might be less intuitive at first glance.
Initialize two number variables.
Apply XOR operations to swap the numbers.
cpp#include<iostream> using namespace std; int main() { int a = 5; int b = 10; // Swap using XOR a = a ^ b; b = a ^ b; a = a ^ b; cout << "After swap, a = " << a << " and b = " << b << endl; return 0; }
XORing
a
andb
stores the result ina
, thenb
is XORed with the newa
to get the originala
, and finally,a
is XORed with the newb
to retrieve the originalb
.
Using Standard C++ Functions
The std::swap()
function from the C++ Standard Library provides a convenient and safe way to swap two variables. This is particularly useful when dealing with complex data types or when you prefer not to handle the swapping logic manually.
Include the
<algorithm>
header that containsstd::swap()
.Use
std::swap()
to swap two variables.cpp#include<iostream> #include<algorithm> using namespace std; int main() { int a = 5; int b = 10; // Swap using std::swap swap(a, b); cout << "After swap, a = " << a << " and b = " << b << endl; return 0; }
This uses the
swap()
function to exchange the values ofa
andb
, simplifying the code and ensuring correct behavior with minimal risk of error. This example demonstrates how to swap two numbers in C++ using a function.
Conclusion
In a C++ program for swapping of two numbers, various methods can be employed, each offering distinct advantages and suitable use cases. From using a simple temporary variable, operating without any additional storage using arithmetic or XOR, to leveraging C++'s standard functions, you should choose the method that best fits your needs and environment. Mastery of these techniques enhances your ability to work effectively with data manipulation in C++, paving the way for more efficient and robust programming.
No comments yet.