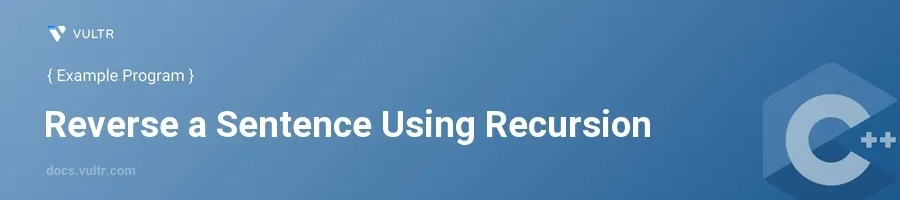
Introduction
Reversing a sentence in C++ using recursion is a common programming task with practical applications in natural language processing, such as reformatting text or preparing input for algorithms that require reversed word order. In this technique, a function calls itself to break the problem into smaller parts, continuing until a base condition is met. This recursive technique is well-suited for sentence reversal, as each function call can handle a segment of the input in a clean and logical manner.
In this article, you will learn how to write a C++ program to reverse a sentence using recursion. Explore several examples that detail each step of the process, ensuring you understand how to apply recursion to reverse sequences of characters efficiently. Whether you want to reverse characters within a string or words within a sentence, recursion in C++ provides a clean and powerful solution.
Understanding Recursion for String Reversal in C++
Theory Behind Recursion
- Recognize how recursion works: Recursion functions by calling itself with a slightly modified parameter until a base condition is met, which stops the recursion.
- Identify the base and recursive cases in reversing a string:
- Base case: If the string is empty, return an empty string.
- Recursive case: Remove the first character, recursively reverse the remainder of the string, then append the removed character at the end.
Write the Base C++ Function
Start with a function prototype: The function, say
reverseSentence
, will take astd::string
as an argument and return astd::string
.Define the base and recursive conditions inside the function.
cpp#include <iostream> #include <string> std::string reverseSentence(const std::string &sentence) { if (sentence.length() == 1) { return sentence; // Base case: a single character string } else { return reverseSentence(sentence.substr(1)) + sentence[0]; // Recursive case } }
In this code snippet, the function checks if the length of the string is
1
, indicating the base case. For the recursive case, the function calls itself with the substring starting from the second character, and the first character is appended after the returned reversed substring.
C++ Programs to Reverse a Sentence Using Recursion
Example 1: Reversing a Simple Sentence
Write a main function to test
reverseSentence
with a simple input.cppint main() { std::string sentence = "Hello World"; std::string reversed = reverseSentence(sentence); std::cout << "Original: " << sentence << std::endl; std::cout << "Reversed: " << reversed << std::endl; return 0; }
This example will output:
Original: Hello World Reversed: dlroW olleH
This demonstrates how each character in the "Hello World" string has been individually reversed using recursion.
Example 2: Handling an Empty String
Modify the main function to test the behavior of
reverseSentence
when provided an empty string.cppint main() { std::string sentence = ""; std::string reversed = reverseSentence(sentence); std::cout << "Original: '" << sentence << "'" << std::endl; std::cout << "Reversed: '" << reversed << "'" << std::endl; return 0; }
The output will be:
Original: '' Reversed: ''
This example shows how the base case effectively handles the situation where no characters are present to reverse.
Example 3: Including Punctuation and Spaces
Test
reverseSentence
with a more complex string that includes punctuation and spaces.cppint main() { std::string sentence = "Hello, World!"; std::string reversed = reverseSentence(sentence); std::cout << "Original: " << sentence << std::endl; std::cout << "Reversed: " << reversed << std::endl; return 0; }
Expect the following output:
Original: Hello, World! Reversed: !dlroW ,olleH
This example confirms that the recursive function deals accurately with punctuation and spaces, keeping their positions relative to the characters reversed.
Conclusion
Reversing a sentence using recursion in C++ elegantly showcases the power of recursive thinking in string manipulation. The examples provided offer a clear foundation for applying this technique to different types of input. Beyond sentence reversal, these concepts extend to other programming challenges where recursion enables clean, concise, and effective solutions. Mastering recursive approaches like this strengthens your algorithmic thinking and equips you to tackle more complex problems with confidence.
No comments yet.