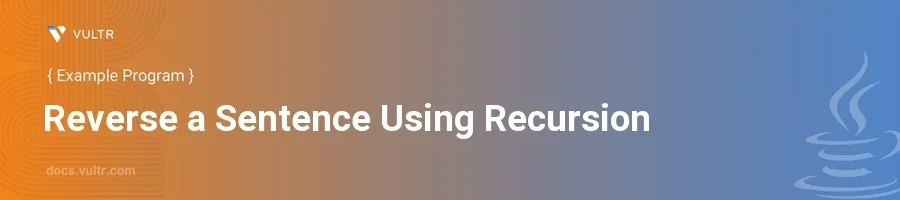
Introduction
Reversing a sentence using recursion in Java illustrates not only the manipulation of strings but also the application of recursion, a fundamental concept in computer science. Recursion involves a method calling itself with modified arguments until it reaches a base case, allowing operations like reversing a sentence to be performed in a very elegant manner.
In this article, you will learn how to effectively reverse a sentence using recursion in Java. Explore detailed examples that demonstrate a recursive approach to this problem, enabling you to understand the nuances of both string manipulation and recursive methods.
Implementing Sentence Reversal Through Recursion
Understanding the Base and Recursive Cases
Before diving into coding, it's crucial to understand the structure of a recursive function for reversing a sentence:
- Base Case: This is the condition under which the recursion stops. For a sentence, the base case is when the length of the sentence is 0 or 1.
- Recursive Case: This involves calling the recursive function with a smaller part of the sentence, gradually reducing its size towards the base case.
Here, the strategy is to handle one word at a time, moving it to the end of the sentence, and then recursively handling the rest of the sentence.
Java Code Example
Here’s how you can implement this in Java:
Define the method
reverseSentence
that takes the sentence to be reversed.Use
.indexOf(' ')
to find the first space (word boundary).Recursively handle the rest of the sentence, moving the first word found to the end until no spaces are left.
javapublic class SentenceReverser { public static String reverseSentence(String sentence) { // Base case: If sentence is empty or has one word, return it if (sentence.isEmpty() || sentence.indexOf(' ') == -1) { return sentence; } int firstSpace = sentence.indexOf(' '); String firstWord = sentence.substring(0, firstSpace); String restOfSentence = sentence.substring(firstSpace + 1); // Recursive case: Reverse the rest of the sentence and add the first word at the end return reverseSentence(restOfSentence) + " " + firstWord; } public static void main(String[] args) { String sentence = "hello world in Java"; String reversed = reverseSentence(sentence); System.out.println("Original: " + sentence); System.out.println("Reversed: " + reversed); } }
In this code:
- The method
reverseSentence
uses recursion to split the sentence into the first word and the remaining sentence. - It checks if the sentence contains spaces. If not, it means the sentence or the remaining part of it is either empty or a single word, which gets returned as is.
- The recursive call processes the remaining sentence and adds the first word at the end.
- The method
Testing the Program
To ensure functionality, test the code with various sentence structures:
- Run the program with different sentences to see how they are reversed.
- Observe the sentence structure, especially with punctuation, to ensure the words are reversed correctly without altering non-space characters.
Conclusion
Reversing a sentence using recursion in Java is an excellent exercise for understanding both string manipulation and the concept of recursion. This method provides a clean and efficient solution to the problem without needing loops or additional data structures like stacks. By mastering recursive methods for such string operations, you enhance your problem-solving skills in Java, making it easier to tackle more complex problems with recursive solutions.
No comments yet.