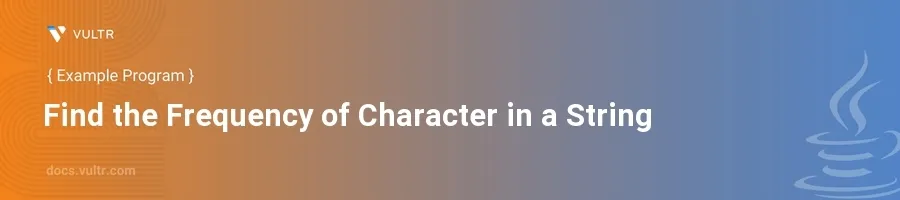
Introduction
Finding the frequency of characters in a string is a common task in programming and data processing, which involves counting how many times each character appears in the string. This can help in various applications such as data validation, cryptography, and text analysis tasks. Java, with its robust standard libraries and string manipulation capabilities, provides efficient methods to accomplish this task.
In this article, you will learn how to determine the frequency of each character in a string using Java. The examples provided will enhance your understanding of string manipulation and character counting in Java, which are fundamental for solving more complex problems in software development.
Using Character Counting with HashMap
Explanation of the Method
- Utilize a
HashMap
to store characters and their corresponding frequencies. - Iterate over each character in the string.
- For each character, update its count in the
HashMap
.
Example Code
Create a Java function to compute the frequency of each character.
Implement the function using a
HashMap
.javaimport java.util.HashMap; import java.util.Map; public class FrequencyFinder { public static void findFrequency(String str) { HashMap<Character, Integer> charCountMap = new HashMap<>(); for (char c : str.toCharArray()) { if (charCountMap.containsKey(c)) { charCountMap.put(c, charCountMap.get(c) + 1); } else { charCountMap.put(c, 1); } } for (Map.Entry<Character, Integer> entry : charCountMap.entrySet()) { System.out.println(entry.getKey() + " " + entry.getValue()); } } public static void main(String[] args) { findFrequency("hello"); } }
This Java code initializes a
HashMap
calledcharCountMap
to store the frequency of each character.The
for
loop iterates over each character in thestr
, and the map is updated accordingly.The second
for
loop prints each character and its frequency.
Using Java 8 Stream API
Explanation of the Method
- Leverage the Stream API for a more concise and functional approach.
- Convert the string into a stream of characters and collect frequencies in a
Map
.
Example Code
Re-write the character frequency function using Java 8 features.
javaimport java.util.stream.Collectors; import java.util.Map; import java.util.function.Function; public class FrequencyFinder { public static void findFrequencyWithStream(String str) { Map<Character, Long> charCountMap = str.chars() .mapToObj(c -> (char) c) .collect(Collectors.groupingBy(Function.identity(), Collectors.counting())); charCountMap.forEach((key, value) -> System.out.println(key + " " + value)); } public static void main(String[] args) { findFrequencyWithStream("hello"); } }
The
chars()
method converts the String into anIntStream
, which is then cast to a stream ofCharacter
.The
collect()
method groups the stream by character, counting occurrences usingCollectors.counting()
.
Conclusion
By mastering the frequency count of characters in a Java string either through a HashMap
or using the Stream API, you enhance your ability to handle string data effectively. Both methods offer flexibility for different scenarios: the HashMap
approach for traditional iterative solutions and the Stream API for a more functional style. Applying these techniques will undoubtedly lead to cleaner, more efficient Java code for various text processing tasks.
No comments yet.