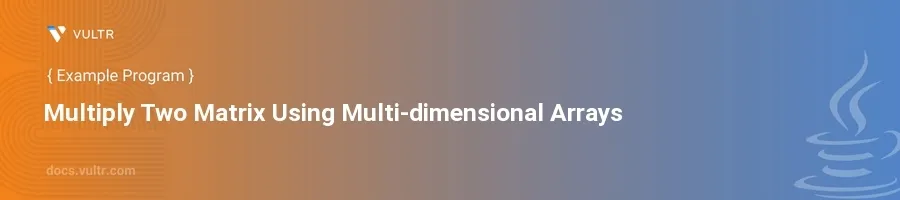
Introduction
Matrix multiplication is a fundamental operation in various scientific computations, graphics, optimizations, and more in programming and mathematics. In Java, this can be efficiently handled using multi-dimensional arrays. Matrix multiplication involves the dot product of rows from the first matrix with columns from the second matrix, and it requires that the number of columns in the first matrix be equal to the number of rows in the second matrix to perform the multiplication.
In this article, you will learn how to multiply two matrices using multi-dimensional arrays in Java. The examples provided will guide you through the process of initializing matrices, performing the multiplication, and displaying the result. This will give you a thorough understanding of handling arrays and implementing matrix arithmetic in Java.
Example of Matrix Multiplication
Initializing the Matrices
Define two matrices as multi-dimensional arrays. Ensure that the number of columns in the first matrix matches the number of rows in the second to perform multiplication.
javaint[][] firstMatrix = { {1, -2, 3}, {4, 0, 6} }; int[][] secondMatrix = { {1, 4}, {-2, 5}, {3, 6} };
This code initializes a 2x3 matrix
firstMatrix
and a 3x2 matrixsecondMatrix
. These dimensions are compatible for multiplication, where the resulting matrix will be 2x2.
Multiplying the Matrices
Determine the dimensions of the resulting matrix, which will have the same number of rows as the first matrix and the same number of columns as the second matrix.
Create a matrix to store the result of the multiplication.
Use three nested loops to multiply the matrices: the outer loop iterates through rows of the first matrix, the middle loop through columns of the second matrix, and the innermost loop performs the multiplication of corresponding elements and sums them up.
javaint[][] resultMatrix = new int[firstMatrix.length][secondMatrix[0].length]; for (int row = 0; row < resultMatrix.length; row++) { for (int col = 0; col < resultMatrix[0].length; col++) { for (int i = 0; i < firstMatrix[0].length; i++) { resultMatrix[row][col] += firstMatrix[row][i] * secondMatrix[i][col]; } } }
In this code snippet,
resultMatrix
stores the product offirstMatrix
andsecondMatrix
. The three nested loops allow each element of the resulting matrix to be computed by summing the products of the corresponding elements of the row from the first matrix and the column from the second matrix.
Displaying the Result
Print the resulting matrix to visualize the product.
javafor (int[] row : resultMatrix) { for (int col : row) { System.out.print(col + " "); } System.out.println(); }
This loop iterates over each row and column in the
resultMatrix
and prints each element, resulting in a formatted output of the matrix.
Conclusion
Mastering matrix multiplication with multi-dimensional arrays in Java enhances your ability to work with complex data structures and algorithms. By utilizing nested loops and understanding array dimensions, you effectively implement crucial mathematical operations. Apply these techniques in problems that involve graphics rendering, scientific computations, and machine learning data preparations. With the foundation laid out in this article, adapt and extend the code for higher-dimensional data or more complex linear algebra operations.
No comments yet.