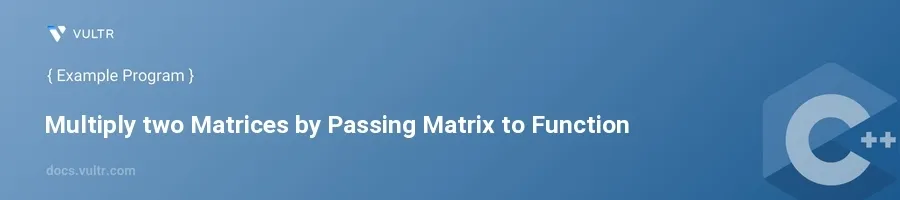
Introduction
Multiplying matrices is a fundamental operation in many computing applications, particularly in fields like graphics, physics simulations, and machine learning. C++ provides a robust platform for executing matrix operations efficiently due to its performance optimizations and low-level control.
In this article, you will learn how to create a C++ program that multiplies two matrices. The approach will involve passing matrices to a function that performs the multiplication, thereby demonstrating not only matrix operations but also the use of functions for structured and reusable code.
Setting Up the Matrix Multiplication Function
In matrix multiplication, you need to ensure that the number of columns in the first matrix matches the number of rows in the second matrix. The resulting matrix will have the number of rows of the first matrix and the number of columns of the second matrix.
Define the Matrix Multiplication Function
Declare a function
multiplyMatrices
that takes the two matrices and their sizes as parameters.Inside the function, implement the matrix multiplication logic.
c++void multiplyMatrices(int firstMatrix[][10], int secondMatrix[][10], int result[][10], int rowFirst, int colFirst, int rowSecond, int colSecond) { if (colFirst != rowSecond) { cout << "Matrix multiplication not possible" << endl; return; } // Initialize the product matrix with 0 for(int i = 0; i < rowFirst; ++i) { for(int j = 0; j < colSecond; ++j) { result[i][j] = 0; } } // Computing product of two matrices for(int i = 0; i < rowFirst; ++i) { for(int j = 0; j < colSecond; ++j) { for(int k = 0; k < colFirst; ++k) { result[i][j] += firstMatrix[i][k] * secondMatrix[k][j]; } } } }
This function checks if matrix multiplication is possible by matching the columns of the first matrix to the rows of the second matrix. It initializes the result matrix and calculates the product by iterating through the matrices and summing the appropriate products.
Displaying the Resulting Matrix
To verify the result, output the matrix to the console.
Print the Resulting Matrix
Create a function
displayMatrix
to print a matrix.Pass the result matrix to this function after multiplication.
c++void displayMatrix(int matrix[][10], int row, int col) { for(int i = 0; i < row; ++i) { for(int j = 0; j < col; ++j) { cout << matrix[i][j] << " "; } cout << endl; } }
This function iterates over the matrix dimensions and outputs each element, formatted in rows and columns.
Main Function to Execute Matrix Multiplication
Tie everything together in the main
function where matrices are defined, multiplied, and displayed.
Initialize matrices and their respective dimensions.
Call
multiplyMatrices
anddisplayMatrix
functions.c++#include <iostream> using namespace std; int main() { int firstMatrix[10][10] = {{1, 2}, {3, 4}}; int secondMatrix[10][10] = {{2, 0}, {1, 2}}; int result[10][10]; int rowFirst = 2, colFirst = 2, rowSecond = 2, colSecond = 2; multiplyMatrices(firstMatrix, secondMatrix, result, rowFirst, colFirst, rowSecond, colSecond); cout << "Product of two matrices is:" << endl; displayMatrix(result, rowFirst, colSecond); return 0; }
Here,
firstMatrix
andsecondMatrix
are defined, andresult
is passed to store the multiplication outcome.rowFirst
andcolFirst
describe the dimensions of the first matrix; similarly,rowSecond
andcolSecond
describe the second matrix.
Conclusion
The C++ program described here effectively demonstrates how to multiply two matrices by passing them to a function. Manage matrix data efficiently using arrays and functions to keep the code clean and maintainable. With these techniques, extend your matrix operations to more complex data manipulation and mathematical computations, enhancing the capability of your applications.
No comments yet.