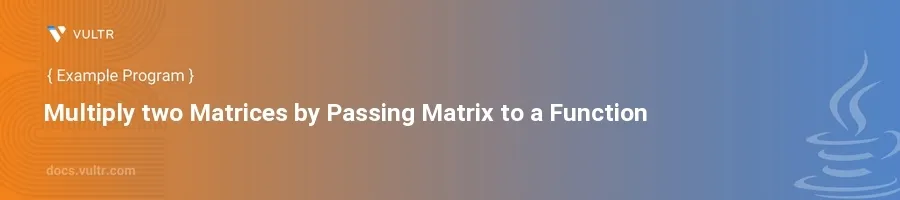
Introduction
Multiplying two matrices in Java involves more than just straightforward arithmetic operations. It requires an understanding of how matrix multiplication works, where each element of the resulting matrix is computed by taking the dot product of row elements from the first matrix with column elements of the second matrix. This operation often finds utilization in various scientific computations, graphics transformations, and programming algorithms.
In this article, you will learn how to multiply two matrices by passing these matrices as arguments to a function in Java. The article will cover the fundamentals of defining matrices, passing them to a method, and using nested loops to compute the resultant matrix. By the end of this guide, you should be able to create a Java function that efficiently multiplies two matrices.
Defining the Matrix Multiplication Function
Setting up the Function
- Start by creating a function that accepts two two-dimensional arrays as its parameters. This function will return a new two-dimensional array as the result of the multiplication.
- Before proceeding with multiplication, check if the number of columns in the first matrix matches the number of rows in the second matrix. If not, multiplication cannot be performed.
Implementing the Multiplication Logic
Define a result matrix. Its size should be rows of the first matrix by columns of the second matrix.
Use nested loops: the outer loop iterates through rows of the first matrix, the middle loop iterates through columns of the second matrix, and the innermost loop performs the multiplication of elements.
javapublic static int[][] multiplyMatrices(int[][] firstMatrix, int[][] secondMatrix) { int r1 = firstMatrix.length; // rows in first matrix int c1 = firstMatrix[0].length; // columns in first matrix (also rows in second matrix) int c2 = secondMatrix[0].length; // columns in second matrix // Resultant matrix dimensions (r1 x c2) int[][] result = new int[r1][c2]; for (int i = 0; i < r1; i++) { for (int j = 0; j < c2; j++) { for (int k = 0; k < c1; k++) { result[i][j] += firstMatrix[i][k] * secondMatrix[k][j]; } } } return result; }
This code defines the matrix multiplication process. The rows and columns of the input matrices determine the loops' structure, ensuring each element of the resulting matrix is calculated by summing the products of the corresponding row and column elements.
Integrating the Function into a Java Program
Creating Sample Matrices
- Define two matrices as two-dimensional arrays and initialize them with some integer values.
Multiplying the Matrices
Call the
multiplyMatrices
function and pass the two matrices initialized earlier.Store the result in a new matrix and print it to display the multiplication result.
javapublic static void main(String[] args) { int[][] firstMatrix = { {1, -2, 3}, {-4, 5, 6}, {7, 8, 9} }; int[][] secondMatrix = { {0, 1, 1}, {1, 0, 1}, {1, 1, 0} }; int[][] result = multiplyMatrices(firstMatrix, secondMatrix); // Printing the result for (int[] row : result) { for (int column : row) { System.out.print(column + " "); } System.out.println(); } }
The main
function initializes two matrices, calls the multiplication function, and prints the resulting matrix. This demonstrates how the function operates in a full Java application.
Conclusion
This tutorial provided a step-by-step approach to implementing a matrix multiplication function in Java. By understanding how to pass matrices to functions and operate on their elements through nested loops, you developed the capability to execute one of the fundamental operations in linear algebra. This practice is beneficial not just for academic purposes but also in practical scenarios like 3D graphics programming and complex mathematical computations. The key is to ensure the logic for matrix dimensions matches to avoid runtime errors during the multiplication process.
No comments yet.