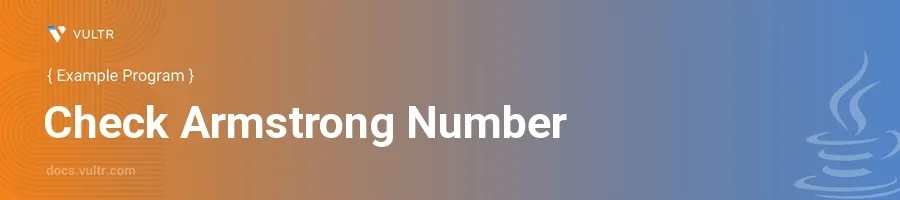
Introduction
An Armstrong number (also known as a narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because (1^3 + 5^3 + 3^3 = 153). This characteristic makes Armstrong numbers particularly interesting from both a mathematical and a programming perspective.
In this article, you will learn how to implement a Java program to check if a given number is an Armstrong number. Using practical examples, gain insights into using loops, conditional statements, and mathematical operations to verify Armstrong numbers effectively.
Java Program to Verify Armstrong Numbers
Determine the Number of Digits
Start by creating a method to calculate the number of digits in the number.
Use a loop to count digits until the number reduces to 0.
javapublic static int countDigits(int number) { int count = 0; while (number != 0) { number /= 10; count++; } return count; }
This method counts how many times the number can be divided by 10 before it becomes zero, effectively counting its digits.
Implement Armstrong Number Check
Develop a method that implements the Armstrong number check using the previously defined digit-count method.
Within this method, decompose the number into its individual digits and compute the power sum.
javapublic static boolean isArmstrong(int number) { int digits = countDigits(number); int originalNumber = number; int sum = 0; while (number != 0) { int remainder = number % 10; sum += Math.pow(remainder, digits); number /= 10; } return sum == originalNumber; }
This method takes each digit, raises it to the total number of digits (computed previously), and sums them. If the sum equals the original number, it's an Armstrong number.
Main Method and Example Checks
Create the main method to run and test whether specific numbers are Armstrong numbers.
Use print statements to display the results.
javapublic class ArmstrongNumberCheck { public static void main(String[] args) { int example1 = 153; int example2 = 9474; int example3 = 123; System.out.println(example1 + " is Armstrong? " + isArmstrong(example1)); System.out.println(example2 + " is Armstrong? " + isArmstrong(example2)); System.out.println(example3 + " is Armstrong? " + isArmstrong(example3)); } }
The example shows how to check for Armstrong numbers using 153, 9474, and 123. The results demonstrate the function's versatility across numbers with varying digits.
Conclusion
Implementing an Armstrong number check in Java helps illustrate essential programming concepts like loops, conditionals, and mathematical operations. With the step-by-step explanation above, test numbers for this intriguing property to deepen understanding of both programming and mathematics. Utilize these examples as a starting point to explore further enhancements, such as creating a GUI or expanding the concept to larger data types like BigInteger
. By leveraging these techniques, optimize and deploy robust Java applications that effectively handle computational tasks.
No comments yet.