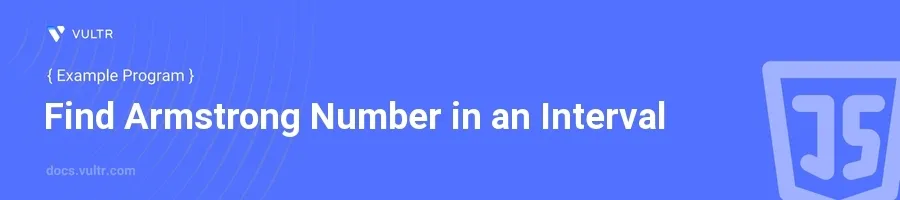
Introduction
An Armstrong number, also known as a narcissistic number, is a number that is the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because (1^3 + 5^3 + 3^3 = 153). These numbers are not just mathematical curiosities; they also find applications in fields like computer science and cryptography.
In this article, you will learn how to create a JavaScript program to identify Armstrong numbers within a specified interval. Follow through specific examples that illustrate how to implement the logic, refine the code, and efficiently find all Armstrong numbers between two given endpoints.
Finding Armstrong Numbers in an Interval
Define the Function to Check Armstrong Numbers
Start by defining a function named
isArmstrong
that checks if a number is an Armstrong number.javascriptfunction isArmstrong(number) { let sum = 0; let temp = number; let digits = number.toString().length; while (temp > 0) { let digit = temp % 10; sum += digit ** digits; temp = Math.floor(temp / 10); } return sum === number; }
This function converts the number to a string to determine the number of digits it contains. It then iterates over each digit, raising it to the power of the total number of digits and accumulating the sum.
Create the Main Function to Find Armstrong Numbers
Develop a function
findArmstrongNumbers
that identifies all Armstrong numbers within a given range.javascriptfunction findArmstrongNumbers(start, end) { let armstrongNumbers = []; for (let num = start; num <= end; num++) { if (isArmstrong(num)) { armstrongNumbers.push(num); } } return armstrongNumbers; }
This function iterates over each number in the specified range and uses the
isArmstrong
function to check if it's an Armstrong number. If it is, the number is added to the result list.
Execute and Test
Test the functions to ensure they work correctly by finding Armstrong numbers in a typical range, for example, from 1 to 500.
javascriptconsole.log(findArmstrongNumbers(1, 500));
This code example checks for any Armstrong numbers between 1 and 500 and logs them to the console.
Conclusion
In this exploration, you learned how to determine Armstrong numbers within a specific range using JavaScript. By deconstructing the problem into manageable parts — identifying Armstrong numbers and then searching within an interval — the JavaScript program presented ensures clarity and efficiency. Use this fundamental concept to further explore patterns or apply similar logic in other programming challenges, enhancing both your problem-solving and coding skills.
No comments yet.