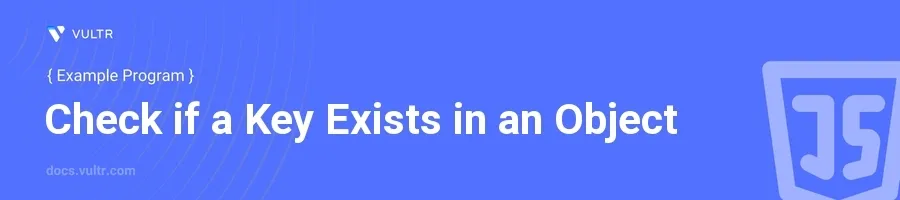
Introduction
Checking if a specific key exists within an object is a common task in JavaScript when dealing with data structures and manipulating object properties. This capability is pivotal for conditionally processing elements of the object without triggering errors due to undefined keys.
In this article, you will learn how to determine if an object contains a certain key using various methods in JavaScript. These methods provide different ways to inspect objects and ensure code functionality by checking properties' presence before accessing their values.
Using the in
Operator
Check Key Presence with in
Create a JavaScript object.
Use the
in
operator to check if a specific key is present in the object.javascriptconst person = { name: "Alice", age: 25 }; const hasName = 'name' in person; console.log(hasName);
The code snippet checks if the key
'name'
exists in the objectperson
. Becauseperson
has a name property, it printstrue
.
Using hasOwnProperty
Method
Verify Key Using hasOwnProperty
Define an object.
Use the
hasOwnProperty
method to examine the presence of a key.javascriptconst car = { make: "Toyota", model: "Camry" }; const hasModel = car.hasOwnProperty('model'); console.log(hasModel);
The
hasOwnProperty
method is used to determine if the objectcar
contains the keymodel
. This method only checks the object's own properties, not inherited ones, hence returningtrue
in this example.
Using Object.keys
and includes
Method
Confirm Key Existence Using includes
Initialize an object.
Employ the combination of
Object.keys
andincludes
to find out if a key exists.javascriptconst book = { title: "1984", author: "George Orwell" }; const keys = Object.keys(book); const containsAuthor = keys.includes('author'); console.log(containsAuthor);
This code first retrieves the keys of the
book
object as an array, and then uses theincludes
method to check if 'author' is one of the keys. The output istrue
, indicating that the key is indeed present.
Conclusion
Determining if a key exists within an object in JavaScript is essential for ensuring error-free code execution, especially when working with dynamic data. Methods like using in
, hasOwnProperty
, and the combination of Object.keys
with includes
provide reliable options for checking the presence of properties. Implement these techniques to improve safety and robustness in your JavaScript code by verifying properties before operating on them.
No comments yet.