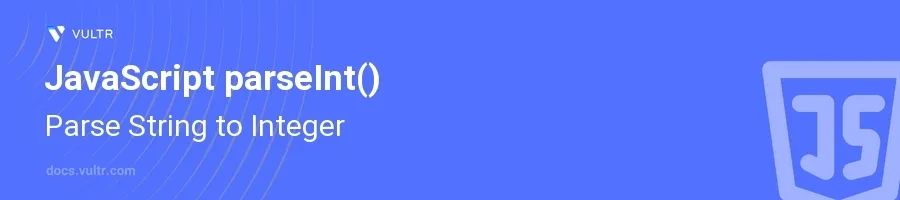
Introduction
The parseInt()
function in JavaScript is a fundamental utility used to convert strings into integers. This method proves invaluable when you need to extract numbers from text data, or when handling inputs that should be integers for mathematical operations. Understanding how to correctly use parseInt()
is crucial when dealing with web forms, server-side validation, or any scenario where type conversion from string to integer is required.
In this article, you will learn how to effectively employ the parseInt()
function in JavaScript. Explore practical examples to grasp how this function handles different string formats and discover how to properly manage unexpected inputs.
Understanding parseInt()
Basic String to Integer Conversion
Start with a simple string that represents a positive integer.
Use
parseInt()
to convert the string to an integer.javascriptvar numberString = "123"; var integer = parseInt(numberString); console.log(integer);
This code converts the string
"123"
into the integer123
. It's a straightforward example of howparseInt()
interprets and converts string data.
Parsing Strings with Leading Characters
Understand that
parseInt()
can handle strings with numbers leading the characters.Implement
parseInt()
on a string that starts with a number followed by characters.javascriptvar mixedString = "456xyz"; var result = parseInt(mixedString); console.log(result);
Here,
parseInt()
successfully extracts456
from the string"456xyz"
. It discards non-numeric characters occurring after the numbers.
Handling Strings with Spaces
Recognize that spaces in strings can affect parsing results.
Invoke
parseInt()
on a string with leading, embedded, or trailing spaces.javascriptvar spacedString = " 789 "; var number = parseInt(spacedString); console.log(number);
With the input
" 789 "
, leading and trailing spaces are ignored, allowingparseInt()
to correctly return the integer789
.
Working with Radix Parameter
Parsing Hexadecimal Strings
Learn how the radix (base) parameter influences the parsing process.
Parse a hexadecimal string using a radix of 16.
javascriptvar hexString = "1A3"; var decimal = parseInt(hexString, 16); console.log(decimal);
This snippet converts the hexadecimal number
"1A3"
to its decimal equivalent,419
, using a radix of16
.
Understanding Radix Defaults
Recognize that the default radix depends on the string format.
See the effect by parsing numbers with different prefixes.
javascriptvar noPrefixNum = "10"; var zeroPrefixNum = "010"; var hexPrefixNum = "0x10"; var normalResult = parseInt(noPrefixNum); var zeroPrefixResult = parseInt(zeroPrefixNum); var hexPrefixResult = parseInt(hexPrefixNum); console.log("No prefix: ", normalResult); // radix 10, returns 10 console.log("Zero prefix: ", zeroPrefixResult); // radix 8 (in some environments), returns 8 console.log("Hex prefix: ", hexPrefixResult); // radix 16, returns 16
In this example, different number representations lead to interpretations with various radix assumptions, resulting in different output values. JavaScript engines may differ in how they handle
0
prefixes.
Conclusion
The parseInt()
function in JavaScript is essential for converting strings to integers in many programming contexts. Its ability to parse integers from both simple and complex string formats makes it a valuable tool in web development, data processing, and anywhere data type conversion is required. Through the examples discussed, you grasp not only the basic usage but also how to handle edge cases and utilize the radix parameter effectively. Use parseInt()
to enhance input validation and data parsing in your JavaScript applications, maintaining precision and reliability in your numerical operations.
No comments yet.