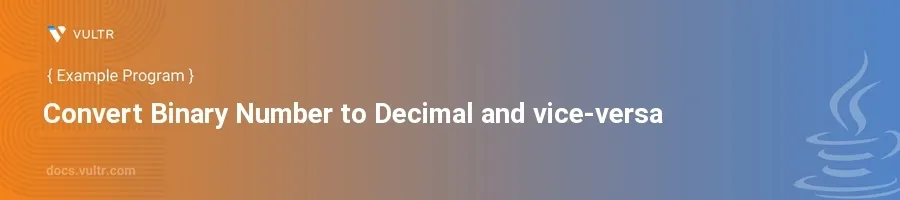
Introduction
Converting numbers between binary and decimal forms is a common task in programming, useful in domains such as computer science and digital electronics. Binary systems (base 2) are pivotal in computing because of their simplicity and efficiency in representation and operations. Conversely, decimal systems (base 10) are used worldwide for general counting and display purposes.
In this article, you will learn how to write Java programs for converting a binary number to a decimal and a decimal number to binary. This exploration will include detailed examples and explanations to ensure you can integrate these conversions into your Java applications effectively.
Converting Binary to Decimal in Java
When converting a binary number to its decimal form, each bit represents an increasing power of 2 from right to left, starting with (2^0). Here’s how to perform this conversion using Java:
Method Description
- Read the binary number as a
String
. - Use the
Integer.parseInt()
method to convert the binaryString
to its decimal form, specifying the base of the number system (which is 2 for binary).
Example Code
```java
public class BinaryToDecimal {
public static void main(String[] args) {
String binaryString = "1101";
int decimal = Integer.parseInt(binaryString, 2);
System.out.println("Decimal Value: " + decimal);
}
}
```
This program converts the binary string "1101" to its decimal equivalent. The Integer.parseInt()
function interprets the string as a base-2 number and converts it to a base-10 integer.
Converting Decimal to Binary in Java
To convert a decimal number to binary, you need to divide the number by 2 and keep track of the remainders. Java simplifies this with the Integer.toBinaryString()
method.
Method Description
- Take a decimal number as input.
- Use
Integer.toBinaryString()
to convert the decimal number to a binary string.
Example Code
```java
public class DecimalToBinary {
public static void main(String[] args) {
int decimal = 13;
String binaryString = Integer.toBinaryString(decimal);
System.out.println("Binary Value: " + binaryString);
}
}
```
This example takes the decimal number 13
and uses Integer.toBinaryString()
to convert and print its binary equivalent. The output will be the string representing the binary number.
Conclusion
Converting between binary and decimal forms in Java is straightforward, thanks to several built-in methods such as Integer.parseInt()
and Integer.toBinaryString()
. These methods not only simplify your code but also ensure accuracy and efficiency in conversions, making them valuable for any Java programmer dealing with numeric data. By incorporating these techniques, you foster a deeper understanding of number systems and enhance your ability to manipulate and represent data within software applications.
No comments yet.