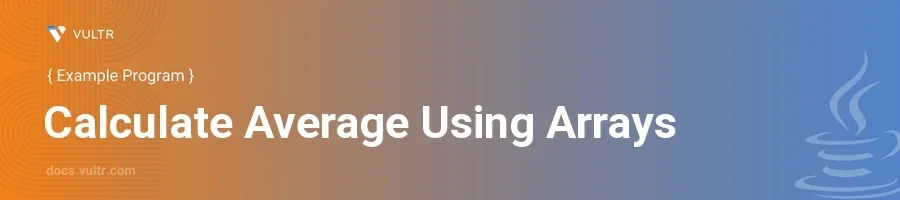
Introduction
Calculating averages is a fundamental task in many programming scenarios, whether in data analysis, school projects, or everyday computing tasks. In Java, one efficient way to calculate an average is by using arrays to store and manipulate numbers. Arrays provide a structured way to manage collections of data, making them ideal for operations such as summing numbers and calculating averages.
In this article, you will learn how to calculate averages using arrays in Java. You'll explore different examples that show how to implement this technique effectively, making your Java programs more efficient and versatile.
Basic Average Calculation
Setup an Array and Calculate the Average
Declare and initialize an array with numeric values.
Compute the sum of the array elements.
Calculate the average by dividing the sum by the array length.
javapublic class AverageCalculator { public static void main(String[] args) { int[] numbers = {10, 20, 30, 40, 50}; // Declare and initialize the array int sum = 0; // Variable to store the sum of array elements for (int num : numbers) { // Enhanced for loop to iterate through the array elements sum += num; // Add each element to sum } double average = (double) sum / numbers.length; // Calculate the average System.out.println("The average is: " + average); // Output the average } }
This code initializes an array of integers, sums the array elements using a for-each loop, and calculates the average. The result is a double value that represents the average of the array elements.
Handling Different Data Types
Calculate the Average for an Array of Doubles
Switch the array data type to double.
Follow similar steps as the previous example, but ensure the variable types match the data type.
javapublic class DoubleAverageCalculator { public static void main(String[] args) { double[] numbers = {10.5, 20.5, 30.5, 40.5, 50.5}; // Array of doubles double sum = 0.0; // Sum variable as double for (double num : numbers) { sum += num; // Accumulate the sum } double average = sum / numbers.length; // Calculate the average System.out.println("The average is: " + average); // Display the average } }
This example shows how to calculate the average when the array elements are type double. It is essential to maintain the type consistency to avoid type mismatch errors.
Advanced Usage: Calculating the Weighted Average
Using Arrays to Store Weights Alongside Values
Create two arrays, one for values and another for their corresponding weights.
Calculate the weighted sum and the sum of weights.
Compute the weighted average.
javapublic class WeightedAverageCalculator { public static void main(String[] args) { double[] values = {50, 60, 70, 80, 90}; // Values double[] weights = {1, 2, 3, 4, 5}; // Corresponding weights double weightedSum = 0; double totalWeight = 0; for (int i = 0; i < values.length; i++) { weightedSum += values[i] * weights[i]; // Calculate the weighted sum totalWeight += weights[i]; // Calculate total weight } double weightedAverage = weightedSum / totalWeight; // Compute weighted average System.out.println("The weighted average is: " + weightedAverage); // Display weighted average } }
This snippet demonstrates how to compute a weighted average, which considers the importance (or weight) of each value. This method is crucial when different elements have varying levels of significance.
Conclusion
Calculating averages using arrays in Java is a versatile technique that can handle different data types and even allow for advanced calculations like weighted averages. By mastering this method, you enhance your ability to carry out statistical computations, improving both the flexibility and effectiveness of your Java applications. Apply these examples in your projects to handle various averaging scenarios effectively.
No comments yet.