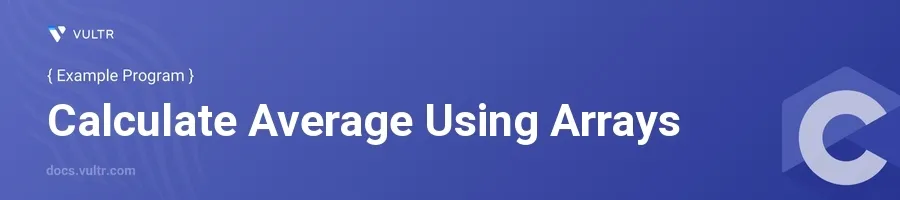
Introduction
Calculating averages is a fundamental operation in many programming tasks, from basic statistics to complex scientific computing. In C, arrays serve as a key data structure to store sequences of elements which can then be manipulated for operations like averaging. C’s straightforward handling of arrays, combined with its control structures, makes it an excellent choice for implementing such algorithms.
In this article, you will learn how to calculate the average in C using arrays. Specifically, we will write a C program to calculate the average of array elements. This tutorial will include setting up the array, using loops to traverse the array, and performing arithmetic to find the average. Examples will be provided to illustrate the process.
C Program to Calculate Average Using Arrays
Initialize the Array and Variables
Start by including the necessary headers and declare the main function.
Initialize the array with the data whose average is to be calculated.
Declare variables needed for the computation, such as accumulators and counters.
c#include <stdio.h> int main() { int numbers[] = {10, 20, 30, 40, 50}; int sum = 0; int count = sizeof(numbers) / sizeof(numbers[0]); double average;
This code snippet initializes an array
numbers
with five integers. Thesum
variable will store the total sum of the array elements, andcount
finds out how many elements there are in the array.
Compute the Sum of the Array Elements
Use a loop to iterate over the array elements.
In each iteration, add the current element to the
sum
.cfor(int i = 0; i < count; i++) { sum += numbers[i]; }
Here, the loop goes through each element in the array
numbers
and adds it tosum
. This step is crucial in finding the sum and average of array elements in C.
Calculate the Average
Divide the total sum by the number of elements to get the average.
Print the calculated average.
caverage = (double)sum / count; printf("Average = %.2f\n", average); return 0; }
Casting
sum
todouble
ensures that the division is performed in floating point arithmetic, preserving any decimal points in the average. The average is then printed to two decimal places.
Using Functions to Calculate the Average in C
Another approach to calculating the average in C is by using a function. This improves modularity and reusability in your program.
Define a function that takes an array and its size as arguments.
Use a loop to compute the sum.
Return the computed average.
c#include <stdio.h> double calculateAverage(int arr[], int size) { // Defining the average function in C int sum = 0; for (int i = 0; i < size; i++) { sum += arr[i]; } return (double)sum / size; // Applying the average formula in C programming } int main() { int numbers[] = {10, 20, 30, 40, 50}; int count = sizeof(numbers) / sizeof(numbers[0]); double avg = calculateAverage(numbers, count); printf("Average = %.2f\n", avg); return 0; }
This method ensures that the function calculateAverage
can be reused for different sets of numbers without modifying the main logic.
Conclusion
Computing the average in C language using arrays is a straightforward process involving initialization, looping through the array to compute a sum, and then performing an arithmetic operation to find the average. This basic pattern is essential in many programming scenarios and provides a foundation for more complex data processing tasks. By following the steps outlined, you have a clear method for calculating average in C that can be adapted and expanded to suit various needs in C programming.
No comments yet.