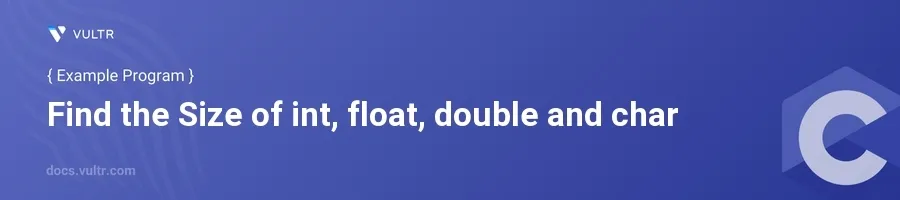
Introduction
Understanding the size of different data types in C, such as int
, float
, double
, and char
, is crucial, especially when dealing with memory-sensitive applications. These sizes can vary depending on the architecture and compiler used, but there are general standards that most compilers follow.
In this article, you will learn how to determine the size of various fundamental data types in C. Examples will demonstrate how to use the sizeof
operator effectively, providing you insight into memory allocation and management in C programming.
Determining the Size of Data Types
Find the Size of an int
Initialize an
int
variable.Use the
sizeof
operator to find its size.c#include <stdio.h> int main() { int integerType; printf("Size of int: %zu bytes\n", sizeof(integerType)); return 0; }
This code outputs the size of an
int
in bytes. The%zu
format specifier is used forsize_t
type returned bysizeof
.
Find the Size of a float
Initialize a
float
variable.Use the
sizeof
operator to determine its size.c#include <stdio.h> int main() { float floatType; printf("Size of float: %zu bytes\n", sizeof(floatType)); return 0; }
This snippet will print the size of a
float
data type in bytes to the console.
Find the Size of a double
Declare a
double
variable.Apply the
sizeof
operator to get the size.c#include <stdio.h> int main() { double doubleType; printf("Size of double: %zu bytes\n", sizeof(doubleType)); return 0; }
Here, the size of a
double
variable in bytes is printed.double
typically requires more memory thanfloat
.
Find the Size of a char
Declare a
char
variable.Use the
sizeof
operator to check its size.c#include <stdio.h> int main() { char charType; printf("Size of char: %zu byte\n", sizeof(charType)); return 0; }
This code confirms that the size of a
char
is 1 byte, which is standard across all standard C compilers.
Conclusion
Using the sizeof
operator in C helps determine the amount of memory needed for storing data types such as int
, float
, double
, and char
. This knowledge is essential for effective memory management and can help in optimizing the performance of applications. By using the examples given, you can apply these techniques to understand and manage memory requirements more accurately in your C programs. This understanding aids in writing more efficient and reliable code.
No comments yet.