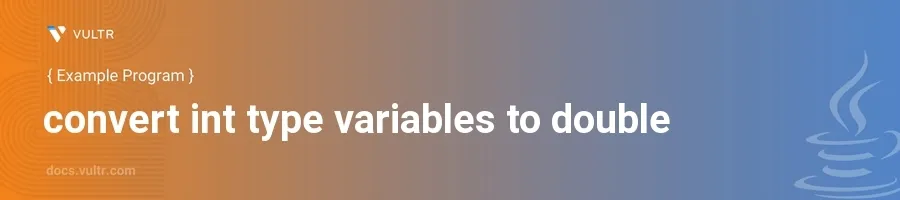
Introduction
In Java, type conversion is a routine operation that often appears when dealing with various data processing activities. This specifically includes converting integers to doubles, which is crucial when ensuring precision in mathematical computations or when interfacing with APIs that expect double values. Converting from an int type to a double type is elegantly handled by Java's automatic type promotion rules, but can also be performed explicitly for clarity and to ensure maintainability in the code.
In this article, you will learn how to convert int type variables to double using a range of examples. Discover the practical usage of both implicit and explicit conversion methods to handle different programming scenarios efficiently.
Implicit Conversion of int to double
Overview of Type Promotion
- Understand that Java automatically converts lower precision types to higher precision types during operations.
- Acknowledge that an int is a 32-bit signed integer while a double is a 64-bit IEEE 754 floating point.
Example: Adding int to a double
Create a simple operation where an int and a double are involved in an arithmetic operation.
javaint integerNumber = 5; double doubleNumber = 6.25; double result = integerNumber + doubleNumber; System.out.println("Result of addition: " + result);
Here,
integerNumber
is automatically converted to a double before the addition operation. This is due to the requirement that both operands need to be of the same type (double), ensuring that there is no loss of precision.
Example: Function Argument Conversion
Create a method that accepts a double parameter and pass an int to this method.
javapublic static double squareRoot(double num) { return Math.sqrt(num); } public static void main(String[] args) { int myInt = 16; double result = squareRoot(myInt); System.out.println("Square root: " + result); }
The
squareRoot
function is designed to take a double, but you can pass an int (myInt
). Java automatically convertsmyInt
from int to double.
Explicit Conversion of int to double
Overview of Explicit Casting
- Learn that explicit casting is necessary when you want to make conversions clear in your code or when dealing with complex data structures and operations where implicit conversion might not occur.
- Remember that in explicit casting, you manually specify the type to convert to.
Example: Manually Casting int to double
Use the casting operator to convert an int to a double explicitly.
javaint integerNumber = 100; double doubleNumber = (double) integerNumber; System.out.println("Explicitly casted double: " + doubleNumber);
Here,
(double)
beforeintegerNumber
is the casting operator that converts the int type explicitly to a double type. This makes the conversion obvious to anyone reading the code.
Example: Using Explicit Casting in Expressions
Incorporate explicit casting in a mathematical expression to control the precision and handling of data types.
javaint num1 = 8; int num2 = 3; double divisionResult = (double) num1 / num2; System.out.println("Result of division: " + divisionResult);
The explicit cast
(double)
is crucial beforenum1
to ensure that the division operation is performed in floating-point precision, yielding a more precise result.
Conclusion
Mastering type conversion in Java is fundamental, particularly when working with numerical computations that require precision and the handling of different data types. Implicit conversions are handy and reduce the code clutter, but explicit conversions are essential when you aim for clarity and explicit control over data types in your code. Utilizing the examples provided, handle int to double conversions confidently in your Java applications, ensuring your operations are precise and your codebase remains robust and understandable.
No comments yet.