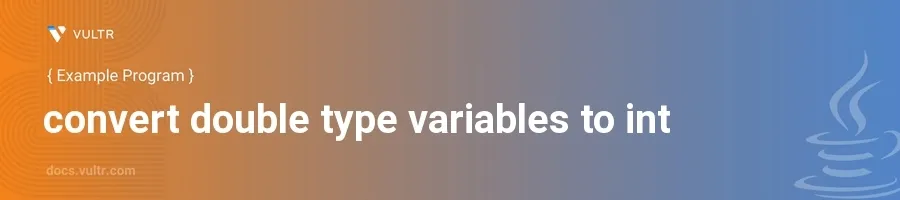
Introduction
In Java, type conversion is a common operation, especially when dealing with numerical data types. Converting from a double to an int is a straightforward task but requires careful handling to avoid loss of information due to truncation. The process of converting a floating-point value (double in this case) to an integer (int) typically involves casting, where the digits after the decimal point are discarded.
In this article, you will learn how to convert double type variables to int in Java using various examples. You will explore the basic casting method and then delve into using different functions like Math.round()
, Math.floor()
, and Math.ceil()
to perform the conversion with specific rounding behaviors.
Basic Type Casting
Type casting in Java is a fundamental concept used to convert a variable from one data type to another. When converting from double to int, the most direct method involves explicit casting, where you tell the compiler to treat a double as an int.
Convert Using Explicit Casting
Declare a double variable.
Cast the double to an int using typical casting syntax.
javadouble myDouble = 9.78; int myInt = (int) myDouble; System.out.println("Converted value: " + myInt);
This snippet directly casts
myDouble
to an int. The decimal part (.78) is truncated, and the result is simply9
.
Using Math Functions for Rounded Conversions
Sometimes, a simple cast isn't sufficient, especially in situations where rounding the double to the nearest integer might be necessary. Java's Math
class provides several methods that can be used for these purposes.
Utilize Math.round()
Apply
Math.round()
to round the double to the nearest long or int.Since
Math.round()
returns long or int based on the method signature, ensure the correct data type is used for the assignment.javadouble myDouble = 9.78; int myInt = (int) Math.round(myDouble); System.out.println("Rounded value: " + myInt);
Here,
Math.round()
accurately rounds9.78
to10
. The double is first converted to the closest long or int, then cast to an int.
Use Math.floor() for Floor Value Conversion
Use
Math.floor()
to get the largest integer less than or equal to the specified double.Cast the result to int to complete the conversion.
javadouble myDouble = 9.78; int myInt = (int) Math.floor(myDouble); System.out.println("Floor value: " + myInt);
Math.floor(myDouble)
returns9.0
. Casting this result to int gives9
, effectively implementing a flooring operation.
Apply Math.ceil() for Ceiling Value Conversion
Implement
Math.ceil()
to find the smallest integer greater than or equal to the given double.Convert the resulting floating point to an int through casting.
javadouble myDouble = 9.12; int myInt = (int) Math.ceil(myDouble); System.out.println("Ceiling value: " + myInt);
Math.ceil(myDouble)
computes to10.0
. When cast to int, the value remains10
, showing ceiling operation.
Conclusion
Converting double to int in Java is an essential skill and can be performed using various methods depending on the specific needs of your application. Starting from simple casts which truncate decimals, to more sophisticated methods using Math.round()
, Math.floor()
, and Math.ceil()
for more controlled rounding, Java offers flexible options to handle double to int conversion. By implementing the techniques discussed, your conversions will be precise, catering to different rounding requirements efficiently. Whether you're working on financial calculations, graphics programming, or any other field dealing with numerical data, these methods ensure your data types are appropriately managed.
No comments yet.