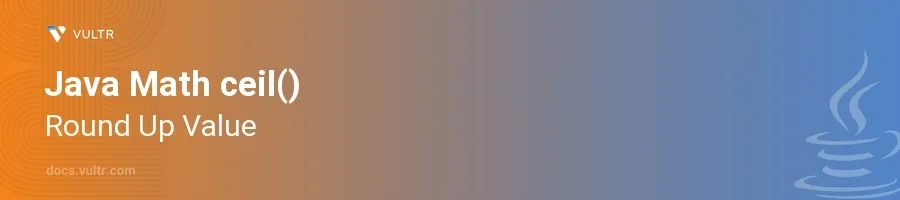
Introduction
The Math.ceil()
method in Java is a widely used function for rounding up numerical values to the nearest larger integer. This utility is particularly useful in scenarios where precision matters, such as financial calculations, graphical computations, or scenario estimations.
In this article, you will learn how to leverage the Math.ceil()
method effectively across different data types and scenarios. Delve into practical applications with detailed examples that highlight the versatility and importance of this function.
Understanding Math.ceil()
Basic Usage with Floating Point Numbers
Consider a basic example where a floating-point number needs rounding up.
Use
Math.ceil()
to round the number to the nearest integer.javadouble value = 3.14; double result = Math.ceil(value); System.out.println(result);
This code rounds the value
3.14
up to4.0
. Even though3.14
is closer to3
than to4
,Math.ceil()
always rounds up.
Rounding Up Negative Numbers
Keep in mind that rounding up negative numbers behaves slightly differently.
Apply
Math.ceil()
on a negative floating-point number.javadouble negativeValue = -1.05; double negativeResult = Math.ceil(negativeValue); System.out.println(negativeResult);
Here, the method rounds
-1.05
to-1.0
. With negative numbers,Math.ceil()
moves the value closer to zero, maintaining its role by moving upwards on the number line.
Handling Whole Numbers and Zero
Recognize that applying
Math.ceil()
to whole numbers and zero results in the same number.Use the method on a whole number and zero to demonstrate this property.
javadouble wholeNumber = 5.0; double zeroValue = 0.0; System.out.println(Math.ceil(wholeNumber)); System.out.println(Math.ceil(zeroValue));
The output for both
Math.ceil(5.0)
andMath.ceil(0.0)
will be5.0
and0.0
, respectively, showing no change as these are already the smallest integers greater than or equal to the values.
Practical Applications of Math.ceil()
Application in Financial Calculations
Use
Math.ceil()
for precise calculation in billing or financial rounding where always rounding up is required.Round up a financial calculation for billing purposes.
javadouble billingAmount = 102.65; double roundedBill = Math.ceil(billingAmount); System.out.println("Rounded billing amount: " + roundedBill);
This ensures that bill amounts are always rounded up to the nearest dollar, a common practice in financial applications to avoid undercharging.
Application in Time Analysis
Consider a scenario in which you need to calculate the number of periods required to cover a certain duration, rounding up to account for any partial period.
Apply
Math.ceil()
to determine the minimum full periods needed.javadouble minutes = 250; double periodLength = 60; double periodsNeeded = Math.ceil(minutes / periodLength); System.out.println("Number of full periods needed: " + periodsNeeded);
This example calculates that
4
full periods (hours in this context) are necessary to cover250
minutes, ensuring that all the time is accounted for.
Conclusion
The Math.ceil()
function in Java is an integral tool for numerically rounding values up to the nearest integer, providing essential utility in a variety of practical applications from financial calculations to time management. Mastering its use enhances your ability to deal with rounding issues comprehensively, ensuring greater accuracy in your programming tasks. By utilizing the examples and information provided, gain confidence in incorporating this method into various Java programming scenarios for improved functionality and precision.
No comments yet.