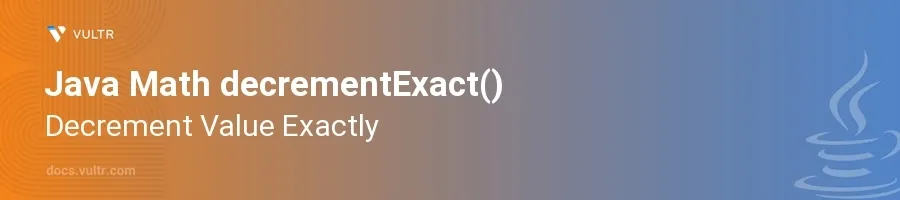
Introduction
The decrementExact()
method in Java is a part of the Math
class, designed to decrement a given number by one. This method ensures that the operation is performed precisely, throwing an exception if the result overflows the data type's limit. It is particularly useful in scenarios where maintaining the accuracy and integrity of numerical calculations is crucial, such as in financial calculations or systems relying on exact values for operation controls.
In this article, you will learn how to utilize the decrementExact()
method efficiently in your Java applications. Explore how this method behaves with different data types and understand how to handle potential overflows effectively.
Understanding decrementExact()
Basic Usage of decrementExact()
Declare a variable and initialize it with a value.
Apply the
decrementExact()
method to decrease the value by one.javaint num = 10; num = Math.decrementExact(num); System.out.println(num); // Output will be 9
This snippet decreases the
num
variable by one usingdecrementExact()
, changing its value from 10 to 9.
Handling Possible Overflows
Recognize that using
decrementExact()
with values at the boundary of their data type might lead to an overflow.Wrap the decrement operation in a try-catch block to handle potential
ArithmeticException
.javatry { int testValue = Integer.MIN_VALUE; testValue = Math.decrementExact(testValue); } catch (ArithmeticException ex) { System.out.println("Overflow occurred: " + ex.getMessage()); }
In this example, attempting to decrement the smallest possible integer value (
Integer.MIN_VALUE
) results in an overflow, which is then caught and reported by theArithmeticException
.
Applying decrementExact() in Real-World Scenarios
Ensuring Data Integrity in Financial Calculations
Use
decrementExact()
in financial systems where accurate decrementing operations are critical.Implement error handling to avoid incorrect results due to overflow.
javalong accountBalance = 5000; try { accountBalance = Math.decrementExact(accountBalance); // Decrement by 1 } catch (ArithmeticException ex) { System.out.println("Transaction error: " + ex.getMessage()); }
Here,
decrementExact()
ensures that the account balance is correctly decremented, and any overflow (though unlikely in this context) is properly managed.
Controlling Loop Variables Precisely
Use
decrementExact()
to control loop decrements where the exact value count matters.Manage looping with precise end conditions to avoid errors.
javaint countdown = 5; try { while (countdown > 0) { System.out.println(countdown); countdown = Math.decrementExact(countdown); } } catch (ArithmeticException ex) { System.out.println("Loop control error: " + ex.getMessage()); }
This code snippet uses
decrementExact()
to decrement a loop counter, ensuring that the countdown is precise and managed correctly without underflowing below 0 unintentionally.
Conclusion
The decrementExact()
function in Java's Math
class provides a powerful tool for accurately decrementing numerical values, crucial in applications where precision is key. By understanding how to use this method correctly and handle potential arithmetic overflows, you can ensure that your Java applications perform reliably and maintain data integrity in critical operations. Employ this function to control numerical operations with confidence in various programming scenarios.
No comments yet.