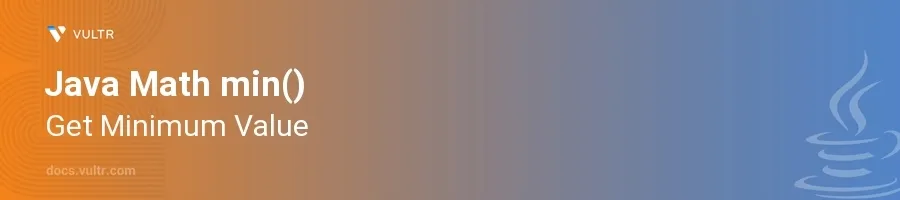
Introduction
The Math.min()
method in Java is crucial for situations where you need to determine the smallest value between two numbers. This function is straightforward and widely used in both simple calculations and complex algorithms to ensure optimal and efficient decision-making based on numeric values.
In this article, you will learn how to effectively utilize the Math.min()
method in Java. Explore the versatility of this function through practical examples that highlight how to compare integer, floating-point numbers, and deal with edge cases in programming scenarios.
Using Math.min() with Integer Values
Compare Two Integer Values
Declare two integer variables.
Apply
Math.min()
to find the smaller value.Output the result.
javaint a = 5; int b = 3; int minimumValue = Math.min(a, b); System.out.println("Minimum value is: " + minimumValue);
This snippet compares integers
a
andb
. TheMath.min()
method identifies3
as the smaller of the two, hence returning it.
Edge Case: Identical Numbers
Consider two identical integer values.
Use
Math.min()
to compare these values.Print the outcome.
javaint c = 7; int d = 7; int resultIdentical = Math.min(c, d); System.out.println("Result with identical numbers: " + resultIdentical);
Even if the numbers are the same,
Math.min()
effectively handles this by returning the value itself, as both are the minimum.
Using Math.min() with Floating-Point Numbers
Compare Two Floating-Point Numbers
Declare two floating-point variables.
Use
Math.min()
to determine the smaller number.Display the result.
javadouble x = 5.75; double y = 3.28; double minimumFloat = Math.min(x, y); System.out.println("Minimum floating-point value is: " + minimumFloat);
Here,
Math.min()
is used to comparex
andy
. The function correctly identifies3.28
as the smaller value.
Handling Negative Values
Include negative floating-point numbers in your example.
Apply
Math.min()
to evaluate.Print the smallest number.
javadouble negative1 = -10.5; double positive1 = 2.3; double minNegative = Math.min(negative1, positive1); System.out.println("Minimum value with a negative is: " + minNegative);
This code snippet showcases how
Math.min()
efficiently sorts between negative and positive numbers, returning-10.5
as the smaller value.
Using Math.min() in Complex Calculations
Determine the Smallest of Computed Values
Use
Math.min()
in an expression involving other mathematical operations.Store the results of these calculations in variables.
Compare the results using
Math.min()
.javaint val1 = 10; int val2 = 20; int squareRootVal1 = (int) Math.sqrt(val1); int tripleVal2 = 3 * val2; int complexMin = Math.min(squareRootVal1, tripleVal2); System.out.println("Smallest in complex calculation: " + complexMin);
In this example, the square root of
val1
and three timesval2
are calculated. TheMath.min()
method then helps determine the smaller outcome.
Conclusion
The Math.min()
function in Java serves as a simple yet powerful tool for determining the minimum value between two inputs. Whether dealing with integers, floating points, handling negative values, or involved in more complex expressions, this method ensures your code handles comparisons cleanly and efficiently. By leveraging the examples provided, harness the full potential of this function to maintain optimal code performance and reliability in various coding environments.
No comments yet.