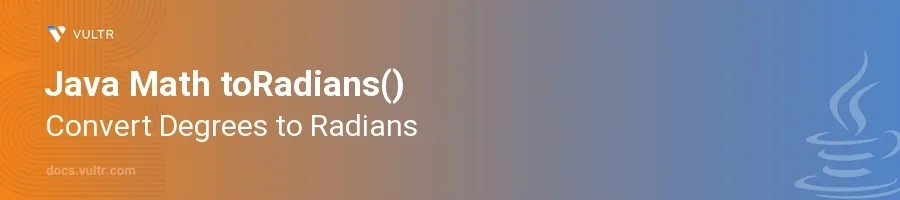
Introduction
The Java Math.toRadians()
method is a simple yet essential tool for converting an angle measured in degrees to an equivalent in radians. This conversion is vital in various fields such as computer graphics, physics simulations, and geometric calculations where radians are the preferred unit of angular measurement due to their natural properties in mathematical expressions.
In this article, you will learn how to use the Java Math.toRadians()
method to convert degrees to radians. Explore practical examples to understand how and where to apply this method effectively in your Java applications.
Understanding Math.toRadians()
Basic Conversion Example
Start by defining a variable that holds an angle in degrees.
Use the
Math.toRadians()
method to convert this degree into radians.Print the result.
javadouble degrees = 180; double radians = Math.toRadians(degrees); System.out.println("Radians: " + radians);
This code converts
180
degrees into radians. The output will show the equivalent radians for180
degrees, which isπ
radians because180
degrees equalsπ
(approximately3.14159
) radians.
Working with Different Degree Values
Prepare a list of various degree values that commonly appear in different applications, such as
0
,30
,45
,90
, and360
degrees.Convert each degree value to radians using a loop.
Print the conversions to observe the results.
javadouble[] degreeValues = {0, 30, 45, 90, 360}; for (double degree : degreeValues) { double radian = Math.toRadians(degree); System.out.println(degree + " degrees is " + radian + " radians"); }
This snippet iteratively converts each degree value in the array
degreeValues
and prints out the degree-radian pairs. It provides a clear visual of how degrees translate to radians in practical scenarios.
Applying the Conversion in a Function
Create a function named
convertToRadians
which takes degrees as an argument and returns the radians.Implement the
Math.toRadians()
inside this function.Call the function with a degree value and display the result.
javapublic static double convertToRadians(double degrees) { return Math.toRadians(degrees); } double degrees = 60; double radians = convertToRadians(degrees); System.out.println("60 degrees is " + radians + " radians");
By defining a dedicated function, integrating degree-to-radian conversion becomes scalable and reusable within larger codebases, making the code clean and maintainable.
Conclusion
The Java Math.toRadians()
function efficiently converts angles from degrees to radians, a conversion crucial for applications requiring precise angular calculations. Familiarize yourself with using this method to ensure accurate results in graphics programming, simulations, and other mathematical computations. With practice, utilize these conversions to enhance the functionality and precision of your Java programs.
No comments yet.