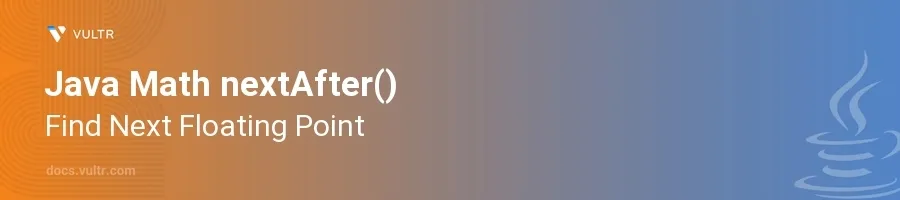
Introduction
The Math.nextAfter()
method in Java is a precise tool for finding the next representable value of a floating-point number in either direction towards a second specified floating-point. This functionality is crucial when performing tasks that require high precision in numerical computations, such as graphics rendering or scientific calculations.
In this article, you will learn how to leverage the Math.nextAfter()
method across various scenarios to control floating-point arithmetic with high precision. Explore how this method behaves with different data types and see practical examples of its application in navigational computational tasks.
Understanding Math.nextAfter()
Basic Usage of nextAfter()
Define two double variables representing the current floating-point value and the direction value.
Use
Math.nextAfter()
to find the next floating-point number.javadouble start = 0.1; double direction = 0.2; double result = Math.nextAfter(start, direction); System.out.println("Next floating point is: " + result);
This code takes a starting value of
0.1
and finds the next floating point number moving towards0.2
. The method returns this adjacent number, which will be displayed.
Negative Direction
Understand that the direction can also be negative.
Call
Math.nextAfter()
with a negative target value to find the previous floating-point number.javadouble start = 0.1; double direction = -0.2; double result = Math.nextAfter(start, direction); System.out.println("Previous floating point is: " + result);
Here, the function computes the previous floating-point number starting from
0.1
and moving towards-0.2
. It effectively decreases the precision moving towards the direction value.
Handling Infinites and NaN
Recognize that
Math.nextAfter()
can handle infinite values and NaN (Not-a-Number).Experiment by using positive infinity, negative infinity, or NaN as direction values.
javadouble start = 0.1; double resultPositiveInf = Math.nextAfter(start, Double.POSITIVE_INFINITY); double resultNegativeInf = Math.nextAfter(start, Double.NEGATIVE_INFINITY); double resultNaN = Math.nextAfter(start, Double.NaN); System.out.println("Towards positive infinity: " + resultPositiveInf); System.out.println("Towards negative infinity: " + resultNegativeInf); System.out.println("Result with NaN direction: " + resultNaN);
This example demonstrates how
Math.nextAfter()
operates when moving towards infinite values or when the direction is undefined (NaN). The method accurately navigates through these special float values.
Practical Applications of Math.nextAfter()
Precision in Graphics Calculations
Apply
Math.nextAfter()
in scenarios such as graphic rendering where precise point calculation is essential.Adjust the float values slightly to ensure no overlap or undesired space between graphical objects.
javadouble position = 0.9999; double adjustedPosition = Math.nextAfter(position, Double.POSITIVE_INFINITY); System.out.println("Adjusted graphical position: " + adjustedPosition);
Adjusting position calculations in graphic rendering can prevent issues like z-fighting or objects flickering due to overlapping coordinates.
Scientific Computation Precision
Use
Math.nextAfter()
in scientific calculators or simulations where precision decimal values are a necessity.Correctly model very small changes in data which can be critical in simulations or calculative models.
javadouble measurement = 0.000000123455; double preciseMeasurement = Math.nextAfter(measurement, Double.POSITIVE_INFINITY); System.out.println("Precise measurement: " + preciseMeasurement);
In scientific computations, achieving ultra-precise results can be crucial, and
Math.nextAfter()
provides the necessary control over floating-point arithmetic.
Conclusion
Use the Math.nextAfter()
method in Java to navigate between floating-point numbers with exceptional precision. This function is indispensable in fields demanding high precision, such as scientific research and graphical computing. By incorporating the Math.nextAfter()
function in your Java applications, ensure calculations are accurate and tailored to your specific needs. Implement these examples to grasp the full potential of floating-point manipulation in your projects.
No comments yet.