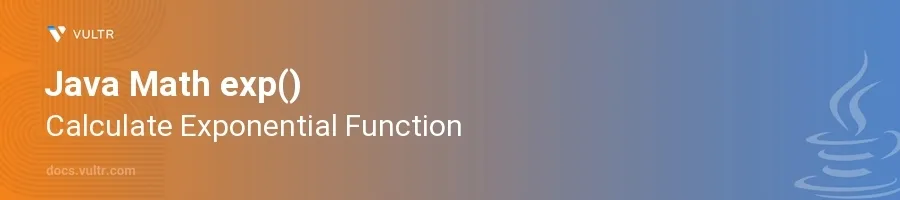
Introduction
The Math.exp()
function in Java is a built-in method used to calculate the exponential of a given number. Specifically, it returns Euler's number e
raised to the power of the specified double value. This function is crucial for scientific calculations that involve exponential growth, decay models, and in various engineering applications where mathematical precision is key.
In this article, you will learn how to effectively use the Math.exp()
method in Java. Explore how to utilize it in different computational scenarios, examine its input and output characteristics, and discover how it interacts with special cases, such as extreme inputs.
Understanding Math.exp() in Java
Basic Usage of Math.exp()
Declare a double variable with a specific value.
Call the
Math.exp()
function with this variable.javadouble value = 2.0; double result = Math.exp(value); System.out.println("Exponential of " + value + " is: " + result);
This code computes the exponential of
2.0
. The result illustrates howMath.exp()
returnse
raised to the power of the input value.
Edge Cases
Explore how Math.exp()
behaves under different edge conditions:
Zero Input: Pass
0
as an argument toMath.exp()
.javadouble resultZero = Math.exp(0); System.out.println("Exponential of 0 is: " + resultZero);
When the input is
0
, the exponential result is1
. This aligns with the mathematical principle that any number raised to the power of zero equals one.Negative Values: Provide a negative number to see how the function computes its exponential.
javadouble negativeValue = -1.0; double resultNegative = Math.exp(negativeValue); System.out.println("Exponential of " + negativeValue + " is: " + resultNegative);
For negative inputs,
Math.exp()
returns a fraction, which represents how rapidly values decay towards zero as the input becomes more negative.Large Positive Values: Use a large positive value to test for overflow scenarios.
javadouble largeValue = 1000.0; double resultLarge = Math.exp(largeValue); System.out.println("Exponential of " + largeValue + " is: " + resultLarge);
Extremely large values illustrate the behavior of
Math.exp()
when approaching the upper limits of double precision. In many cases, this might result in positive infinity due to overflow.Double Infinity and NaN: Experiment with special floating-point values like
Double.POSITIVE_INFINITY
andDouble.NaN
.javaSystem.out.println("Exponential of Positive Infinity: " + Math.exp(Double.POSITIVE_INFINITY)); System.out.println("Exponential of NaN: " + Math.exp(Double.NaN));
When
Math.exp()
processesDouble.POSITIVE_INFINITY
, it returnsDouble.POSITIVE_INFINITY
. ProcessingDouble.NaN
similarly returnsDouble.NaN
, maintaining the properties of non-numbers throughout mathematical operations.
Conclusion
The Math.exp()
function in Java is a powerful tool for computing the exponential of numbers, essential in various mathematical, scientific, and engineering computations. From handling standard numbers, navigating edge cases, to processing extreme and special float values, Math.exp()
proves reliable and consistent. Gain familiarity with these techniques, and integrate them into solutions that require exponential calculations to enhance the precision and effectiveness of your computational tasks.
No comments yet.