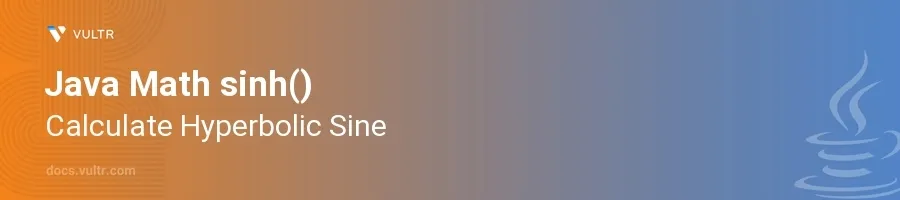
Introduction
The Math.sinh()
method in Java is used to calculate the hyperbolic sine of a double value. This function is an essential part of the Java Math class, aiding in various mathematical calculations involving hyperbolic functions, commonly used in engineering, physics, and hyperbolic geometry.
In this article, you will learn how to effectively use the Math.sinh()
method to compute the hyperbolic sine values in Java. Explore the method's applications with practical examples and understand its behavior with different inputs to enhance your mathematical computations in Java programming.
Understanding Math.sinh()
Syntax and Parameters
- Familiarize yourself with the syntax of
Math.sinh()
. The method takes a single parameter:double x
: The number whose hyperbolic sine is to be calculated.
Basic Usage
Calculate the hyperbolic sine of a number. To find the hyperbolic sine of
x
, use:javadouble x = 3.0; double result = Math.sinh(x); System.out.println("Hyperbolic Sine of " + x + " is: " + result);
This code calculates the hyperbolic sine of
3.0
. TheMath.sinh()
function computes the result based on the formula ( \sinh(x) = \frac{e^x - e^{-x}}{2} ).
Edge Case Considerations
Consider special values input like zero, positive infinity, and negative infinity.
Apply
Math.sinh()
to these edge cases.javaSystem.out.println("sinh(0): " + Math.sinh(0)); System.out.println("sinh(Double.POSITIVE_INFINITY): " + Math.sinh(Double.POSITIVE_INFINITY)); System.out.println("sinh(Double.NEGATIVE_INFINITY): " + Math.sinh(Double.NEGATIVE_INFINITY));
- For
sinh(0)
, the output is0.0
as the hyperbolic sine of zero is zero. - For
sinh(Double.POSITIVE_INFINITY)
, it returnsDouble.POSITIVE_INFINITY
indicating the function's handling of infinity inputs. - For
sinh(Double.NEGATIVE_INFINITY)
, the result isDouble.NEGATIVE_INFINITY
.
- For
Practical Applications
Calculating Time Dilation in Special Relativity
Use
Math.sinh()
in formulas to compute real-world physics problems, like time dilation.Implement the formula using
Math.sinh()
.javadouble velocity = 0.7; // relative velocity (c = 1) double gamma = Math.sinh(velocity / Math.sqrt(1 - velocity * velocity)); System.out.println("Lorentz factor (Gamma) is: " + gamma);
This snippet uses
Math.sinh()
in the calculation of the Lorentz factor (Gamma), which is used to determine the amount of time dilation according to the theory of relativity.
Conclusion
The Math.sinh()
method in Java is a versatile tool for calculating the hyperbolic sine of a number. It handles a wide range of values smoothly, from zero to positive and negative infinity. Use this function in a variety of scientific, engineering, and mathematical applications to produce accurate and efficient results. By leveraging the examples and explanations provided, enhance your toolkit for performing complex mathematical calculations in Java.
No comments yet.