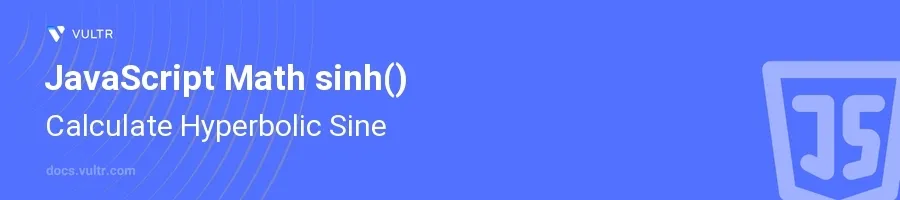
Introduction
The JavaScript Math object offers a variety of functions that support complex mathematical calculations, integral to any computation-heavy JavaScript application. One such function is Math.sinh()
, which calculates the hyperbolic sine of a number. This function is paramount in fields involving engineering, physics, or any mathematical modeling that involves hyperbolic functions.
In this article, you will learn how to utilize the Math.sinh()
function to compute the hyperbolic sine of different numbers, showcasing its application across various numerical inputs including zero, positive numbers, and negative numbers.
Calculating Hyperbolic Sine with Math.sinh()
Understanding the Function
Math.sinh()
computes the hyperbolic sine of a given number x
using the formula:
[ \sinh(x) = \frac{e^x - e^{-x}}{2} ]
Where ( e ) is Euler's number, approximately equal to 2.71828.
Examples of Using Math.sinh()
Calculate Hyperbolic Sine of Zero
Pass zero to the
Math.sinh()
function and observe the output.javascriptconst resultZero = Math.sinh(0); console.log(resultZero);
This will output
0
, as hyperbolic sine of zero is zero.
Calculate Hyperbolic Sine of a Positive Number
Use
Math.sinh()
with a positive argument to compute its hyperbolic sine.javascriptconst positiveNumber = 2; const resultPositive = Math.sinh(positiveNumber); console.log(resultPositive);
This calculates the hyperbolic sine of 2, which should output a non-zero value close to 3.6268.
Calculate Hyperbolic Sine of a Negative Number
Apply
Math.sinh()
on a negative number to see the result.javascriptconst negativeNumber = -1; const resultNegative = Math.sinh(negativeNumber); console.log(resultNegative);
The result will be approximately
-1.1752
, displaying the hyperbolic sine of -1.
Handling Large Numbers
When working with significantly large values, keep in mind that JavaScript might produce results close to Infinity due to Math.sinh()
’s exponential nature. Here's an example:
Compute the hyperbolic sine of a large number.
javascriptconst largeNumber = 20; const resultLarge = Math.sinh(largeNumber); console.log(resultLarge);
This code snippet will likely output a value that approaches JavaScript's Infinity, emphasizing caution during such calculations.
Conclusion
The Math.sinh()
function in JavaScript is an important tool for computing the hyperbolic sine, a fundamental mathematical function used in various scientific and engineering calculations. By integrating this function into your JavaScript applications, you enable precise and efficient computations necessary for any tasks involving hyperbolic sine evaluations. Whether dealing with physics problems, engineering models, or simply exploring mathematical concepts, Math.sinh()
provides robust support for nuanced arithmetic operations. Remember to handle large input values judiciously to avoid unexpected overflows resulting in Infinity.
No comments yet.