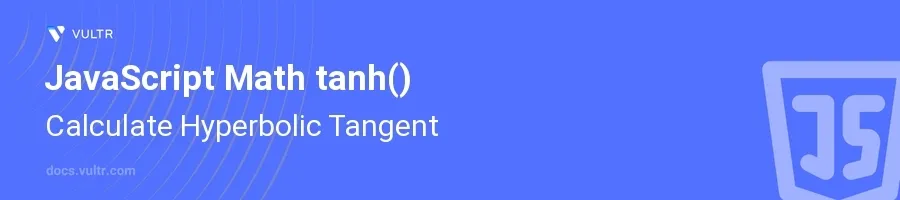
Introduction
The Math.tanh()
function in JavaScript is an essential method used to compute the hyperbolic tangent of a number. This mathematical function is particularly useful in various fields, including physics, engineering, and especially in machine learning algorithms where activation functions are pivotal. Understanding how to implement and leverage this function can enhance the performance and accuracy of such algorithms.
In this article, you will learn how to effectively use the Math.tanh()
method in JavaScript. Explore practical examples that demonstrate how to calculate the hyperbolic tangent of different numbers and understand the behavior of this function across various input values.
Using Math.tanh() in JavaScript
Basic Usage of Math.tanh()
Understand that
Math.tanh(x)
returns the hyperbolic tangent of a numberx
.Utilize this method to calculate the hyperbolic tangent of zero.
javascriptconst tanhZero = Math.tanh(0); console.log(tanhZero); // Output will be 0
This code snippet calculates the hyperbolic tangent of
0
, which mathematically results in0
.
Positive and Negative Values
Recognize that the hyperbolic tangent function is odd, meaning
Math.tanh(-x) === -Math.tanh(x)
.Calculate the hyperbolic tangent of positive and negative values to observe this behavior.
javascriptconst tanhPositive = Math.tanh(1); const tanhNegative = Math.tanh(-1); console.log(tanhPositive); // Output will be approximately 0.7616 console.log(tanhNegative); // Output will be approximately -0.7616
Here, the outputs demonstrate the odd nature of the hyperbolic tangent function. The negative input yields the negative of the hyperbolic tangent of the corresponding positive input.
Larger Values
Note that as the absolute value of
x
increases,Math.tanh(x)
approaches1
or-1
.Calculate the hyperbolic tangent for larger absolute values.
javascriptconst tanhLargePositive = Math.tanh(10); const tanhLargeNegative = Math.tanh(-10); console.log(tanhLargePositive); // Output will be very close to 1 console.log(tanhLargeNegative); // Output will be very close to -1
This demonstrates that the
Math.tanh()
function tends to1
as the input value increases and-1
as it decreases. This is useful in situations like neural networks where output needs to be bounded.
Conclusion
The Math.tanh()
function in JavaScript is a powerful tool for computing the hyperbolic tangent of given numbers. It finds significant applications in scientific computing, engineering, and various types of neural network calculations where hyperbolic functions are used as activation functions to model complex relationships. By learning to utilize this function, you expand your toolkit in JavaScript, enabling efficient and effective mathematical computations critical for high-performance applications. Employ this function judiciously in scenarios where its unique properties bring the most benefit.
No comments yet.