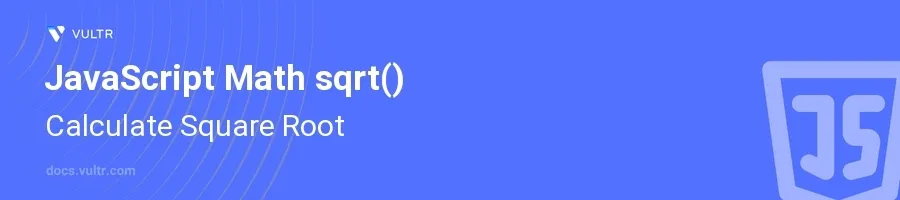
Introduction
The Math.sqrt()
function in JavaScript is a standard method used to calculate the square root of a number. This built-in function is part of the Math object and is widely used in mathematical calculations, especially in fields like engineering, physics, and computer graphics where calculations involving distances or quadratic equations are common.
In this article, you will learn how to use the Math.sqrt() function effectively in JavaScript. You will explore various examples that demonstrate how to calculate square root in javascript, handle negative numbers, and integrate this function into more complex mathematical formulas.
Understanding Math.sqrt() in JavaScript
Basic Square Root Calculation
Start with a positive number for which you want to find the square root.
Use
Math.sqrt()
to calculate the square root.javascriptlet number = 9; let result = Math.sqrt(number); console.log(result); // Outputs: 3
This code calculates the square root of
9
, which is3
.
Handling Non-Numeric or Undefined Values
Pass a non-numeric value or
undefined
toMath.sqrt()
to see the result.Note that JavaScript handles these gracefully, turning them into NaN (Not a Number) or using type coercion if possible.
javascriptlet invalidNumber = "test"; let undefinedNumber; console.log(Math.sqrt(invalidNumber)); // Outputs: NaN console.log(Math.sqrt(undefinedNumber)); // Outputs: NaN
Here, both non-numeric and undefined values result in
NaN
when passed toMath.sqrt()
.
Advanced Usage of Math.sqrt()
Calculating Hypotenuse Using Pythagorean Theorem
Define two sides of a right triangle.
Use
Math.sqrt()
in conjunction withMath.pow()
to calculate the hypotenuse.javascriptlet a = 3; let b = 4; let hypotenuse = Math.sqrt(Math.pow(a, 2) + Math.pow(b, 2)); console.log(hypotenuse); // Outputs: 5
Using the Pythagorean theorem, the hypotenuse of a right triangle with sides
3
and4
is5
.
Working with Negative Numbers
Understand that attempting to find the square root of a negative number directly with
Math.sqrt()
returnsNaN
.Implement checks or use complex numbers library to handle such cases if needed.
javascriptlet negativeNumber = -16; let sqrtNegative = Math.sqrt(negativeNumber); console.log(sqrtNegative); // Outputs: NaN
Math.sqrt()
does not support square root calculations for negative numbers directly, resulting inNaN
.
How to Do Square Root in JavaScript
In JavaScript, you can calculate the square root of a number using the Math.sqrt() function. Here’s an example.
let num = 25;
let sqrtValue = Math.sqrt(num);
console.log(sqrtValue);
Conclusion
The Math.sqrt()
function in JavaScript is a powerful and easy-to-use tool for performing square root calculations. Whether handling basic arithmetic or integrating this function into complex mathematical formulas, Math.sqrt()
offers precise and reliable results. Remember its limitations with negative numbers and non-numeric values, and ensure your data inputs are prepared accordingly for effective usage. Whether developing games, scientific simulations, or everyday calculations, Math.sqrt()
ensures your JavaScript code handles square root calculations efficiently.
No comments yet.