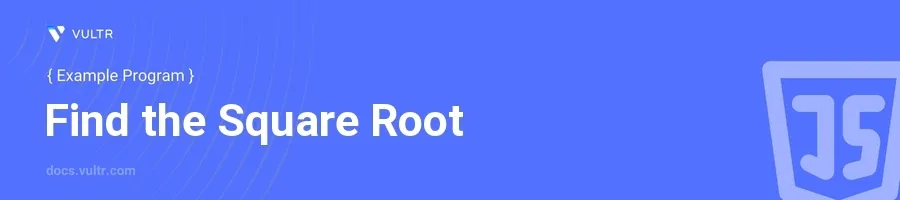
Introduction
Finding the square root of a number is a common operation in many computing scenarios, from basic arithmetic to advanced mathematical computations in various applications. JavaScript, being a versatile scripting language, provides straightforward methods to perform this task efficiently.
In this article, you will learn how to calculate the square root of a number using JavaScript. This includes using the built-in Math library, handling edge cases, and ensuring your code is robust and user-friendly through practical examples.
Using the Math.sqrt() Method
The Math.sqrt()
function in JavaScript is used to calculate the square root of a number. This built-in method returns the square root of a specified number, and will return NaN
if the number is negative or not a number.
Calculate Square Root of a Positive Number
To find the square root of a positive number:
Define a positive number.
Apply the
Math.sqrt()
function and display the result.javascriptlet number = 16; let squareRoot = Math.sqrt(number); console.log(squareRoot); // Outputs: 4
Here,
Math.sqrt(number)
calculates the square root of 16, which is 4. This method is simple and effective for straightforward square root calculations.
Handle Non-Numeric Inputs
To ensure the function handles non-numeric and negative inputs properly:
Create a function that checks if the input is a non-negative number.
Use
Math.sqrt()
to calculate the square root if the input is valid.Return an appropriate message if the input is invalid.
javascriptfunction findSquareRoot(input) { if (typeof input !== 'number' || input < 0) { return 'Please enter a non-negative number'; } return Math.sqrt(input); } console.log(findSquareRoot(25)); // Outputs: 5 console.log(findSquareRoot(-1)); // Outputs: Please enter a non-negative number
This function first verifies the input to be a number and non-negative. If the input fails the check, it informs the user to provide a valid number.
Dealing with Zero and Infinity
Handling zero and infinity as inputs:
Understand the behavior of
Math.sqrt()
for special cases.Run examples to observe the outputs for zero and infinity.
javascriptconsole.log(Math.sqrt(0)); // Outputs: 0 console.log(Math.sqrt(Infinity)); // Outputs: Infinity
In JavaScript, the square root of zero is zero, and the square root of infinity is infinity. These edge cases are handled elegantly by
Math.sqrt()
.
Conclusion
Using Math.sqrt()
in JavaScript allows you to effectively calculate the square root of numbers including handling of special cases like non-numeric values, zero, and infinity. By leveraging the built-in Math library, ensure your applications can perform this mathematical computation reliably and efficiently. Apply these techniques to enhance numeric processing tasks in your projects, ensuring they cater to a broad range of input scenarios.
No comments yet.